Assignment 1 (Robots)
Submit on the CMS two weeks into the course
Monitoring Self Replicating Robots
Introduction
People
have been thinking about the idea of self-replicating robots for a very
long time. Science fiction author Philip K. Dick wrote a short story featuring
self-replicating robots titled Autofac in 1955. The idea was even featured
in the 1921 play R.U.R. by Czech playwright Karel Čapek, from which
we get the word "robot" itself (see the OED).
However, self-replicating robots are not solely in the domain of science
fiction. Cornell's own Hod Lipson proved that it was possible for robots
to build copies of themselves in a 2005 project (http://www.news.cornell.edu/stories/May05/selfrep.ws.html,
be sure to check out the video).
Today, robots build many of the things we use every day, from the chips in this computer, to the car Dr. Gries drives (http://www.roboticsonline.com/public/articles/archivedetails.cfm?id=485). It is not hard to imagine that one day robots will build robots.
Your task
in this assignment is to develop a Java class Robot,
which will maintain information about robots capable of building other
robots, and a JUnit class RobotTester to
maintain a suite of testcases for Robot. This
assignment will help illustrate how Java’s classes and objects can
be used to maintain data about a collection of things —like robots.
Read the whole assignment before starting. Follow the instructions given below in order to complete the assignment as quickly and as efficiently as possible.
HELP
If you don't know where to start, if you don't understand testing, if you are lost, SEE SOMEONE IMMEDIATELY —the course instructor, a TA, a consultant. Do not wait. A little one-on-one help can do wonders.
Class Robot
An instance of class Robot represents a single robot. It has several fields that one might use to describe a robot, as well as methods that operate on these fields. Here are the fields, all of which should be private (you can choose the names of these fields).
- serial number (an int: a number used to identify this robot)
- size (a char —'L' for large or 'S' for small)
- month of construction (an int)
- year of construction (an int)
- maker (a Robot —the name on the tab of folder of the Robot object that built this one)
- number of robots built by this one (an int)
- name (a String, if not specified otherwise this is the same as the serial number, but a String instead of an int)
Here are some details about these fields.
The name is a String of characters. Two robots may have the same name just as two people may have the same name. If a robot has not been assigned a name, its name should be the same as its serial number.
- The month of construction is in the range 1..12, representing a month from January to December. The year of construction is something like 1997 or 2012. Do not worry about invalid dates; do not write code that checks whether dates are valid: assume they are valid.
- The serial number will be a unique identifier for each Robot. Assume all tags given are unique; do not write code to check whether a robot with a given serial number already exists.
- The maker is the name of a Robot object (manila folder) that built this robot. It is not a String. The maker is null if it is either not known or if this robot was not built by other robots.
Accompanying the declarations of these fields should be comments that describe what each field means –what it contains. For example, on the declaration of the field size, state in a comment that the field represents the size of a robot, 'L' for large, etc. The collection of the comments on these fields is called the class invariant. The class describes all legal values for the fields together.
Whenever you write a method (see below), look through the class invariant and convince yourself that class invariant is correct when the method terminates.
Robot Methods
Class Robot has the following methods. Pay close attention to the parameters and return values of each method. The descriptions, while informal, are complete.
Constructor | Description |
---|---|
Robot(int serialNum, int month, int year, char size) | Constructor: a new Robot. Parameters are, in order,
the serial number (> 0) of this robot, the month and year of construction,
and size: 'L' (large) or 'S' (small). The new
robot's name is the same as its serial number and it was not made by
another robot. It has made no robots. Precondition: all parameters are valid as described. |
Robot(String name, int serialNum, char size, Robot maker, int month, int year) | Constructor: a new Robot. Parameters
are, in order, the name of the Robot, its serial number (> 0),
its size —'L' (large) or 'S' (small)—
, its maker, and the month and year of construction. Precondition: maker is not null, and all parameters are valid as described. |
When you write a constructor body, be sure to set all the fields to appropriate values.
Getter Method | Description |
---|---|
getSerialNum() | = this robot's serial number (an int) |
getName() | = the name of this robot (a String) |
getSize() | = the size of this robot ('L' for large or 'S' for small) (a char) |
getMonth() | = the month this robot was constructed, in the range 1..12 (an int) |
getYear() | = the year this robot was built (an int) |
getMaker() | = the name (of the folder containing) the robot that built this robot (a Robot) |
getNumberProduced() | = how many robots this robot has built (an int) |
toString() | = a String representation of this Robot. Its precise specification is discussed below. |
Setter Method | Description |
---|---|
setSerialNum(int i) | Set this robot’s serial number to i (this has no effect on the robot's name). Precondition: i > 0. |
setName(String n) | Set the name of this robot to n. |
setSize(char s) | Set the type of this robot to s ('L' for large, 'S' for small). Precondition: s is either 'L' or 'S' |
setMonth(int m) | Set the month this robot was built to m. Precondition: m is in the range 1..12 |
setYear(int y) | Set the year this robot was built to y. |
setMaker(Robot r) | Set the predecessor of this robot to r. Precondition: This robot’s maker is null, and r is not null. |
Comparison Method | Description |
---|---|
isOlder(Robot r) | = "r is not null, and this robot is older than r —i.e. this robot was built before r". (a boolean) |
isOlder(Robot r1, Robot r2 ) | Static method. = "r1 and r2 are not null, and r1 is older than r2 —i.e. r1 was built before r2". (a boolean) |
Make sure that the names of your methods match those listed above exactly, including capitalization. The number of parameters and their order must also match. The best way to ensure this is to copy and paste our names. Our testing will expect those method names, so any mismatch will fail during our testing. Parameter names will not be tested —you can change the parameter names if you want.
Each method MUST be preceded by an appropriate specification, or "spec", as a Javadoc comment. The best way to ensure this is to copy and paste. After you have pasted, be sure to do any necessary editing. For example, the spec of a function does not have to say that the function yields a boolean or int or anything else, because that is known from the header of the method.
A precondition should not be tested by the method; it is the responsibility of the caller to ensure that the precondition is met. For example, it is a mistake to call method setMaker with null for the maker argument. However, in functions isOlder, the tests for r, r1 and r2 not null MUST be made (because they have no precondition).
It is possible for robot r1 to be r2's maker and visa versa, at the same time. We do not check for such strange occurrences.
In writing methods setMaker, be careful! If M is becoming the maker of this Robot, then M has built one more robot, and its field that contains the number of robots it built has to be updated accordingly.
Do not use if-statements, and you may only use conditional expression (...?... :...) in function toString. Boolean expressions, using the operators && (AND), || (OR), and ! (NOT), are sufficient to implement the comparison methods.
Function toString
We illustrate the required format of the output of toString with an example and then make some comments. Here is an example of output of toString:
"42: small robot Arthur. Built 7/2005. Built 2 other robots."
Here are some points about this output.
- Exactly one blank separates each piece of information, and the periods and colon are necessary.
- The number at the beginning is this robot's serial number.
- The entire word "large" or "small" appears, not just the character 'L' or 'S'.
- The name of the robot follows the word "robot" even if it is the same as its serial number.
- The maker is not described in the output of this function.
- If the robot has built exactly 1 other robot, the word "robot" should appear instead of "robots".
- In writing the body of function toString, do not use if-statements or switches. Instead, use the conditional expression (condition ? expression1: expression2) This is the only place you can use the conditional expression.
Class RobotTester
We require you to build a suite of test cases as you develop class Robot in a JUnit class RobotTester. Make sure that your test suite adheres to the following principles:
- Every method in your class Robot needs at least one test case in the test suite.
- The more interesting or complex a method is, the more test cases you should have for it. What makes a method 'interesting' or complex can be the number of interesting combinations of inputs that method can have, the number of different results that the method can have when run several times, the different results that can arise when other methods are called before and after this method, and so on.
- Here is one important point. If an argument of a method can be null, there should be a test case that has null for that argument.
- Test each method (or group of methods) as soon as you finish, before moving on to the rest of the assignment.
How to do this assignment
First, remember that if-statements are not allowed. If your assignments has if-statements, you will be asked to revise the assignment and resubmit.
Second, you should develop and test the class in a methodologically sound way, which we outline below. If we detect that you did not develop it thus, we may ask you to start from scratch and write one of the other alternatives for this assignment.
- First, start a new folder on your hard drive that will contain the files for this project. Start every new project in its own folder.
- Second, write a class Robot using DrJava. In it, declare the fields in class Robot, compiling often as you proceed. Write comments that specify what these fields mean.
- Third, after finishing the first section, start a new JUnit class, calling it RobotTester.
- Fourth, this assignment should properly be done in several parts. For each part, write the methods, write a test procedure in class RobotTester and add test cases to it for all the methods you wrote, and then test the methods thoroughly. Do not go on to the next group of methods until the ones you are working on are correct. If we determine that you did not follow this way of doing things —for example, you wrote all methods and then tried to test, we may ask you to set this assignment aside and do another instead. Here are the groups of methods.
- (1) The first constructor and all the getter methods of class Robot.
- (2) The second constructor.
- (3) Function toString.
- (4) The setter methods.
- (5) The two comparison methods.
At each step, make sure all methods are correct before proceeding to the next step. When adding a new method, cut and paste the comment and the header from the assignment handout and then edit the comment. It must have suitable javadoc specifications as well as suitable comments on the field declarations. The assignment will not be accepted for testing until it does.
Other hints and directions
- Do not use if statements.
- Remember that a String literal is enclosed in double quotation marks and a char literal is enclosed in single quotation marks.
- Be sure to create the javadoc specification and look at it carefully, to be sure that the methods are specified thoroughly and clearly.
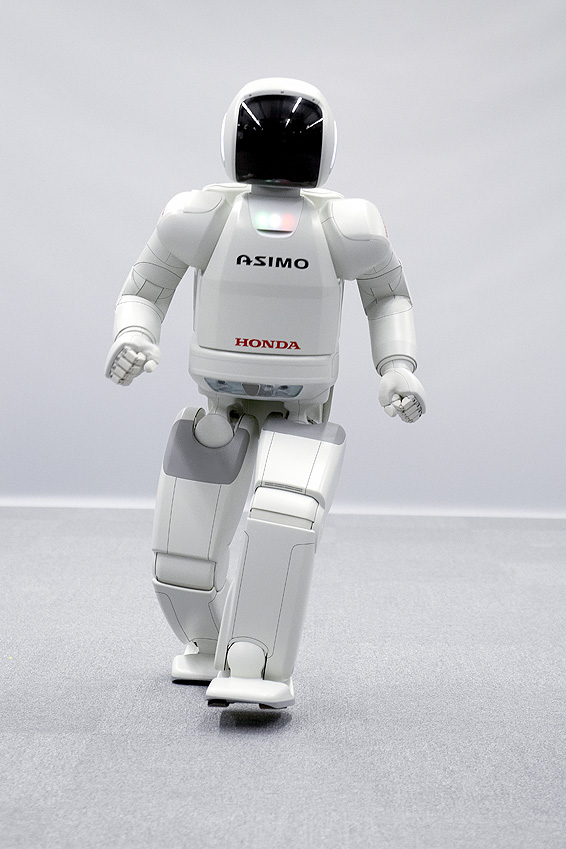
Procedure for submission
You may submit the assignment whenever you wish. We will look at it in several steps.
1. If the field specifications and the javadoc specifications are not appropriate, we will ask you to fix them and resubmit.
2. If the field and javadoc specs are ok. We will look at your test cases. If they are inadequate, we will ask you to fix them and resubmit.
3. If the test cases are adequate, we will test your program. If there are errors, you will be asked to correct them and resubmit.
Only when the assignment passes all three categories, will it be accepted as complete.
Submitting the assignment
First, at the top of file Robot.java, put a comment that says that you looked carefully at the specifications produced by clicking the javadoc button and checked that the specifications of methods and the class specification were OK (put this comment after doing what the comments says).
Second, upload files Robot.java and RobotTester.java on the CMS. You will be asked to upload Ass1.java and Ass1Tester.java. Don't worry about this; just upload files Robot.java and RobotTester.java. Make sure you submit .java files. do not submit a file with the extension/suffix .java~. You can ensure that you do this by setting your preferences in your operating system so that extensions always appear.