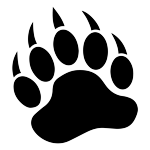 |
CUGL 1.3
Cornell University Game Library
|
42 #include <cugl/util/CUDebug.h>
43 #include "CUMathBase.h"
68 #if defined CU_MATH_VECTOR_SSE
70 __attribute__((__aligned__(16)))
struct {
82 #elif defined CU_MATH_VECTOR_NEON64
84 __attribute__((__aligned__(16)))
struct {
131 #pragma mark Constructors
147 #if defined CU_MATH_VECTOR_SSE
148 v = _mm_set_ps(
w,
z,
y,
x);
149 #elif defined CU_MATH_VECTOR_NEON64
152 this->x =
x; this->y =
y; this->z =
z; this->w =
w;
164 std::memcpy(
this, array, 4*
sizeof(
float));
174 #if defined CU_MATH_VECTOR_SSE
175 v = _mm_sub_ps(p2.v,p1.v);
176 #elif defined CU_MATH_VECTOR_NEON64
177 v = vsubq_f32(p2.v,p1.v);
179 x = p2.
x-p1.
x;
y = p2.
y-p1.
y;
z = p2.
z-p1.
z;
w = p2.
w-p1.
w;
210 #if defined CU_MATH_VECTOR_SSE
211 v = _mm_set_ps(
w,
z,
y,
x);
212 #elif defined CU_MATH_VECTOR_NEON64
215 this->x =
x; this->y =
y; this->z =
z; this->w =
w;
230 std::memcpy(
this, array, 4*
sizeof(
float));
242 #if defined CU_MATH_VECTOR_SSE || defined CU_MATH_VECTOR_NEON64
259 #if defined CU_MATH_VECTOR_SSE
260 v = _mm_sub_ps(p2.v,p1.v);
261 #elif defined CU_MATH_VECTOR_NEON64
262 v = vsubq_f32(p2.v,p1.v);
264 x = p2.
x-p1.
x;
y = p2.
y-p1.
y;
z = p2.
z-p1.
z;
w = p2.
w-p1.
w;
275 #if defined CU_MATH_VECTOR_SSE
276 v = _mm_setzero_ps();
277 #elif defined CU_MATH_VECTOR_NEON64
278 v = vmovq_n_f32(0.0f);
287 #pragma mark Static Arithmetic
419 #pragma mark Arithmetic
441 return Vec4(clampf(
x,min.
x,max.
x), clampf(
y,min.
y,max.
y),
442 clampf(
z,min.
z,max.
z), clampf(
w,min.
w,max.
w));
453 #if defined CU_MATH_VECTOR_SSE
454 this->v = _mm_add_ps(this->v,v.v);
455 #elif defined CU_MATH_VECTOR_NEON64
456 this->v = vaddq_f32(this->v,v.v);
458 x += v.
x;
y += v.
y;
z += v.
z;
w += v.
w;
474 #if defined CU_MATH_VECTOR_SSE
475 this->v = _mm_add_ps(this->v,_mm_set_ps(
w,
z,
y,
x));
476 #elif defined CU_MATH_VECTOR_NEON64
477 float32x4_t tmp = {
x,
y,
z,
w};
478 this->v = vaddq_f32(this->v,tmp);
480 this->x +=
x; this->y +=
y; this->z +=
z; this->w +=
w;
493 #if defined CU_MATH_VECTOR_SSE
494 this->v = _mm_sub_ps(this->v,v.v);
495 #elif defined CU_MATH_VECTOR_NEON64
496 this->v = vsubq_f32(this->v,v.v);
498 x -= v.
x;
y -= v.
y;
z -= v.
z;
w -= v.
w;
514 #if defined CU_MATH_VECTOR_SSE
515 this->v = _mm_sub_ps(this->v,_mm_set_ps(
w,
z,
y,
x));
516 #elif defined CU_MATH_VECTOR_NEON64
517 float32x4_t tmp = {
x,
y,
z,
w};
518 this->v = vsubq_f32(this->v,tmp);
520 this->x -=
x; this->y -=
y; this->z -=
z; this->w -=
w;
533 #if defined CU_MATH_VECTOR_SSE
534 v = _mm_mul_ps(v,_mm_set1_ps(s));
535 #elif defined CU_MATH_VECTOR_NEON64
536 v = vmulq_n_f32(v,(float32_t)s);
538 x *= s;
y *= s;
z *= s;
w *= s;
554 #if defined CU_MATH_VECTOR_SSE
555 v = _mm_mul_ps(v,_mm_set_ps(sw, sz, sy, sx));
556 #elif defined CU_MATH_VECTOR_NEON64
557 float32x4_t tmp = {sx, sy, sz, sw};
558 v = vmulq_f32(v,tmp);
560 x *= sx;
y *= sy;
z *= sz;
w *= sw;
573 #if defined CU_MATH_VECTOR_SSE
574 this->v = _mm_mul_ps(this->v,v.v);
575 #elif defined CU_MATH_VECTOR_NEON64
576 this->v = vmulq_f32(this->v,v.v);
578 x *= v.
x;
y *= v.
y;
z *= v.
z;
w *= v.
w;
602 Vec4&
divide(
float sx,
float sy,
float sz,
float sw);
621 #if defined CU_MATH_VECTOR_SSE
622 v = _mm_sub_ps(_mm_setzero_ps(), v);
623 #elif defined CU_MATH_VECTOR_NEON64
624 v = vsubq_f32(vmovq_n_f32(0.0f), v);
681 x = func(
x);
y = func(
y);
z = func(
z);
w = func(
w);
696 return Vec4(func(
x), func(
y), func(
z), func(
w));
701 #pragma mark Operators
781 return result.
add(v);
821 return result.
scale(s);
836 return result.
scale(v);
870 #pragma mark Comparisons
982 bool equals(
const Vec4& v,
float variance=CU_MATH_EPSILON)
const;
985 #pragma mark Linear Attributes
1025 bool isUnit(
float variance=CU_MATH_EPSILON)
const {
1027 return fabsf(
dot) <= variance;
1104 #if defined CU_MATH_VECTOR_SSE
1105 return _mm_dp_ps(v,v,0xF1)[0];
1113 #pragma mark Linear Algebra
1206 return other * (
dot(other)/other.
dot(other));
1218 float dw = (
w == 0 ? 1 : 1/
w);
1252 *
this *= (1.f - alpha);
1253 return *
this += other * alpha;
1269 return *
this * (1.f - alpha) + other * alpha;
1284 void smooth(
const Vec4& target,
float elapsed,
float response);
1288 #pragma mark Static Linear Algebra
1379 #pragma mark Conversion Methods
1391 std::string
toString(
bool verbose =
false)
const;
1450 operator Vec2()
const;
1480 operator Vec3()
const;
1529 #pragma mark Friend Operations
1538 inline const Vec4 operator*(
float x,
const Vec4& v) {
1540 return result.scale(x);
Vec4 & operator*=(float s)
Definition: CUVec4.h:731
static Vec4 * subtract(const Vec4 &v1, const Vec4 &v2, Vec4 *dst)
bool operator<(const Vec4 &v) const
Vec4 getLerp(const Vec4 &other, float alpha)
Definition: CUVec4.h:1268
Vec4 getProjection(const Vec4 &other) const
Definition: CUVec4.h:1205
Vec4 & map(std::function< float(float)> func)
Definition: CUVec4.h:680
Vec4 & subtract(const Vec4 &v)
Definition: CUVec4.h:492
static const Vec4 HOMOG_ORIGIN
Definition: CUVec4.h:122
bool operator>(const Vec4 &v) const
float z
Definition: CUVec4.h:102
bool over(const Vec4 &v) const
bool under(const Vec4 &v) const
Vec4 & scale(float sx, float sy, float sz, float sw)
Definition: CUVec4.h:553
bool operator>=(const Vec4 &v) const
float lengthSquared() const
Definition: CUVec4.h:1103
float distance(const Vec4 &v) const
Definition: CUVec4.h:1060
static float angle(const Vec4 &v1, const Vec4 &v2)
static Vec4 * divide(const Vec4 &v, float s, Vec4 *dst)
static const Vec4 UNIT_X
Definition: CUVec4.h:112
static const Vec4 ONE
Definition: CUVec4.h:110
static const Vec4 UNIT_Z
Definition: CUVec4.h:116
Vec4 & set(float x, float y, float z, float w)
Definition: CUVec4.h:209
Vec4(const Vec4 &p1, const Vec4 &p2)
Definition: CUVec4.h:173
Definition: CUColor4.h:1084
static Vec4 * reciprocate(const Vec4 &v, Vec4 *dst)
static Vec4 * scale(const Vec4 &v, float s, Vec4 *dst)
float y
Definition: CUVec4.h:100
bool isHomogenous() const
Definition: CUVec4.h:1035
Vec4 & add(float x, float y, float z, float w)
Definition: CUVec4.h:473
Vec4 & operator=(const float *array)
Definition: CUVec4.h:195
float dot(const Vec4 &v) const
Definition: CUVec4.h:1121
const Vec4 operator*(float s) const
Definition: CUVec4.h:819
Vec4 getReciprocal() const
Definition: CUVec4.h:665
Vec4 getHomogenized()
Definition: CUVec4.h:1233
Vec4 & operator*=(const Vec4 &v)
Definition: CUVec4.h:742
Vec4 getMap(std::function< float(float)> func) const
Definition: CUVec4.h:695
Vec4 & setZero()
Definition: CUVec4.h:274
static const Vec4 UNIT_W
Definition: CUVec4.h:118
Vec4 & operator/=(const Vec4 &v)
Definition: CUVec4.h:766
float w
Definition: CUVec4.h:104
void smooth(const Vec4 &target, float elapsed, float response)
Vec4 getNegation() const
Definition: CUVec4.h:649
Vec4 getMidpoint(const Vec4 &other) const
Definition: CUVec4.h:1178
bool operator!=(const Vec4 &v) const
bool isNearZero(float variance=CU_MATH_EPSILON) const
Definition: CUVec4.h:1000
Vec4 & project(const Vec4 &other)
Definition: CUVec4.h:1191
bool operator<=(const Vec4 &v) const
Vec4 & scale(float s)
Definition: CUVec4.h:532
static const Vec4 ZERO
Definition: CUVec4.h:108
Vec4(float x, float y, float z, float w)
Definition: CUVec4.h:146
Definition: CUColor4.h:73
static const Vec4 HOMOG_X
Definition: CUVec4.h:124
Vec4 & set(const float *array)
Definition: CUVec4.h:229
bool operator==(const Vec4 &v) const
Vec4(const float *array)
Definition: CUVec4.h:163
Vec4 & set(const Vec4 &v)
Definition: CUVec4.h:241
Vec4 & operator+=(const Vec4 &v)
Definition: CUVec4.h:709
Vec4 getClamp(const Vec4 &min, const Vec4 &max) const
Definition: CUVec4.h:440
const Vec4 operator-(const Vec4 &v) const
Definition: CUVec4.h:793
bool isUnit(float variance=CU_MATH_EPSILON) const
Definition: CUVec4.h:1025
Vec4()
Definition: CUVec4.h:136
std::string toString(bool verbose=false) const
static Vec4 * midpoint(const Vec4 &v1, const Vec4 &v2, Vec4 *dst)
Vec4 cross(const Vec4 &v)
Definition: CUVec4.h:1135
static const Vec4 HOMOG_Y
Definition: CUVec4.h:126
Vec4 & add(const Vec4 &v)
Definition: CUVec4.h:452
const Vec4 operator-() const
Definition: CUVec4.h:805
static Vec4 * add(const Vec4 &v1, const Vec4 &v2, Vec4 *dst)
Vec4 & scale(const Vec4 &v)
Definition: CUVec4.h:572
Vec4 & operator/=(float s)
Definition: CUVec4.h:753
Vec4 & negate()
Definition: CUVec4.h:620
Vec4 & homogenize()
Definition: CUVec4.h:1217
static const Vec4 UNIT_Y
Definition: CUVec4.h:114
float length() const
Definition: CUVec4.h:1087
Vec4 & set(const Vec4 &p1, const Vec4 &p2)
Definition: CUVec4.h:258
bool isInvertible() const
const Vec4 operator/(float s) const
Definition: CUVec4.h:848
Vec4 & operator-=(const Vec4 &v)
Definition: CUVec4.h:720
float getAngle(const Vec4 &other) const
const Vec4 operator+(const Vec4 &v) const
Definition: CUVec4.h:779
static Vec4 * clamp(const Vec4 &v, const Vec4 &min, const Vec4 &max, Vec4 *dst)
float x
Definition: CUVec4.h:98
bool equals(const Vec4 &v, float variance=CU_MATH_EPSILON) const
const Vec4 operator/(const Vec4 &v) const
Definition: CUVec4.h:863
static Vec4 * negate(const Vec4 &v, Vec4 *dst)
static const Vec4 HOMOG_Z
Definition: CUVec4.h:128
Vec4 & subtract(float x, float y, float z, float w)
Definition: CUVec4.h:513
Vec4 getNormalization() const
Definition: CUVec4.h:1164
Vec4 & lerp(const Vec4 &other, float alpha)
Definition: CUVec4.h:1251
float distanceSquared(const Vec4 &v) const
const Vec4 operator*(const Vec4 &v) const
Definition: CUVec4.h:834