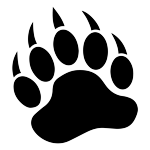 |
CUGL 1.3
Cornell University Game Library
|
45 #ifndef __CU_TWO_ZERO_FIR_H__
46 #define __CU_TWO_ZERO_FIR_H__
48 #include <cugl/math/dsp/CUIIRFilter.h>
49 #include <cugl/math/CUMathBase.h>
50 #include <cugl/util/CUAligned.h>
107 #pragma mark SPECIALIZED FILTERS
137 void stride(
float gain,
float* input,
float* output,
size_t size,
unsigned channel);
161 void single(
float gain,
float* input,
float* output,
size_t size);
186 void dual(
float gain,
float* input,
float* output,
size_t size);
214 void trio(
float gain,
float* input,
float* output,
size_t size);
239 void quad(
float gain,
float* input,
float* output,
size_t size);
264 void quart(
float gain,
float* input,
float* output,
size_t size);
270 #pragma mark Constructors
297 TwoZeroFIR(
unsigned channels,
float b0,
float b1,
float b2);
318 #pragma mark IIR Signature
356 void setCoeff(
const std::vector<float> &bvals,
const std::vector<float> &avals);
369 const std::vector<float>
getBCoeff()
const;
382 const std::vector<float>
getACoeff()
const;
384 #pragma mark Specialized Attributes
398 void setBCoeff(
float b0,
float b1,
float b2);
427 void setNotch(
float frequency,
float radius );
435 void setZeroes(
float zero1,
float zero2);
437 #pragma mark Filter Methods
454 void step(
float gain,
float* input,
float* output);
475 void calculate(
float gain,
float* input,
float* output,
size_t size);
490 size_t flush(
float* output);
const std::vector< float > getACoeff() const
const std::vector< float > getBCoeff() const
void setCoeff(const std::vector< float > &bvals, const std::vector< float > &avals)
void setBCoeff(float b0, float b1, float b2)
unsigned getChannels() const
Definition: CUTwoZeroFIR.h:327
Definition: CUTwoZeroFIR.h:91
void setNotch(float frequency, float radius)
void setChannels(unsigned channels)
static bool VECTORIZE
Definition: CUTwoZeroFIR.h:268
void step(float gain, float *input, float *output)
void setZeroes(float zero1, float zero2)
size_t flush(float *output)
void calculate(float gain, float *input, float *output, size_t size)