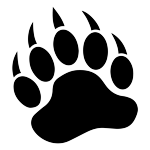 |
CUGL 1.3
Cornell University Game Library
|
34 #ifndef __CU_TOUCHSCREEN_H__
35 #define __CU_TOUCHSCREEN_H__
38 #include <cugl/math/CURect.h>
43 #define CU_INVALID_TOUCH -1
48 typedef Sint64 TouchID;
122 #pragma mark Listeners
198 #pragma mark Constructors
217 virtual void dispose()
override;
219 #pragma mark Data Polling
300 const std::vector<TouchID>
touchSet()
const;
302 #pragma mark Listeners
455 #pragma mark Input Device
487 virtual void queryEvents(std::vector<Uint32>& eventset)
override;
bool touchDown(TouchID touch) const
Definition: CUTouchscreen.h:231
const ContactListener getEndListener(Uint32 key) const
const std::vector< TouchID > touchSet() const
bool addMotionListener(Uint32 key, MotionListener listener)
virtual void dispose() override
bool touchPressed(TouchID touch) const
Definition: CUTouchscreen.h:242
Vec2 touchPosition(TouchID touch) const
Definition: CUTimestamp.h:61
bool removeEndListener(Uint32 key)
virtual void clearState() override
bool isListener(Uint32 key) const
virtual bool updateState(const SDL_Event &event, const Timestamp &stamp) override
bool removeBeginListener(Uint32 key)
Vec2 touchOffset(TouchID touch) const
const ContactListener getBeginListener(Uint32 key) const
Timestamp timestamp
Definition: CUTouchscreen.h:56
std::function< void(const TouchEvent &event, const Vec2 &previous, bool focus)> MotionListener
Definition: CUTouchscreen.h:183
std::unordered_map< Uint32, ContactListener > _beginListeners
Definition: CUTouchscreen.h:192
TouchID touch
Definition: CUTouchscreen.h:58
TouchEvent(TouchID finger, const Vec2 &point, float force, const Timestamp &stamp)
Definition: CUTouchscreen.h:77
std::unordered_map< TouchID, Vec2 > _previous
Definition: CUTouchscreen.h:187
bool touchReleased(TouchID touch)
Definition: CUTouchscreen.h:258
virtual ~Touchscreen()
Definition: CUTouchscreen.h:210
bool addBeginListener(Uint32 key, ContactListener listener)
std::function< void(const TouchEvent &event, bool focus)> ContactListener
Definition: CUTouchscreen.h:151
std::unordered_map< TouchID, Vec2 > _current
Definition: CUTouchscreen.h:189
std::unordered_map< Uint32, ContactListener > _finishListeners
Definition: CUTouchscreen.h:194
Touchscreen()
Definition: CUTouchscreen.h:205
bool addEndListener(Uint32 key, ContactListener listener)
virtual void queryEvents(std::vector< Uint32 > &eventset) override
const MotionListener getMotionListener(Uint32 key) const
std::unordered_map< Uint32, MotionListener > _moveListeners
Definition: CUTouchscreen.h:196
Definition: CUTouchscreen.h:53
Vec2 position
Definition: CUTouchscreen.h:60
TouchEvent()
Definition: CUTouchscreen.h:67
unsigned int touchCount() const
Definition: CUTouchscreen.h:293
float pressure
Definition: CUTouchscreen.h:62
bool removeMotionListener(Uint32 key)
virtual bool requestFocus(Uint32 key) override
Definition: CUTouchscreen.h:120