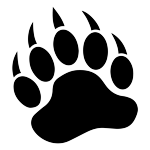 |
CUGL 1.3
Cornell University Game Library
|
33 #ifndef _CU_TEXTURE_H__
34 #define _CU_TEXTURE_H__
36 #include <cugl/math/CUMathBase.h>
37 #include <cugl/math/CUSize.h>
54 class Texture :
public std::enable_shared_from_this<Texture> {
110 std::shared_ptr<Texture> _parent;
128 #pragma mark Constructors
183 bool initWithData(
const void *data,
int width,
int height,
206 #pragma mark Static Constructors
222 static std::shared_ptr<Texture>
alloc(
int width,
int height,
224 std::shared_ptr<Texture> result = std::make_shared<Texture>();
244 static std::shared_ptr<Texture>
allocWithData(
const void *data,
int width,
int height,
246 std::shared_ptr<Texture> result = std::make_shared<Texture>();
269 static std::shared_ptr<Texture>
allocWithFile(
const std::string& filename) {
270 std::shared_ptr<Texture> result = std::make_shared<Texture>();
271 return (result->initWithFile(filename) ? result :
nullptr);
307 #pragma mark Attributes
327 return (_parent !=
nullptr ? _parent->isActive() : _active);
339 return (_parent !=
nullptr ? _parent->hasMipMaps() : _hasMipmaps);
368 void setName(std::string name) { _name = name; }
378 const std::string&
getName()
const {
return _name; }
420 return (_parent !=
nullptr ? _parent->getMinFilter() : _minFilter);
432 return (_parent !=
nullptr ? _parent->getMagFilter() : _magFilter);
463 return (_parent !=
nullptr ? _parent->getWrapS() : _wrapS);
474 return (_parent !=
nullptr ? _parent->getWrapT() : _wrapT);
493 #pragma mark Atlas Support
501 const std::shared_ptr<Texture>&
getParent()
const {
return _parent; }
510 std::shared_ptr<Texture>
getParent() {
return _parent; }
531 std::shared_ptr<Texture>
getSubTexture(GLfloat minS, GLfloat maxS, GLfloat minT, GLfloat maxT);
585 #pragma mark Rendering
601 #pragma mark Conversions
612 std::string
toString(
bool verbose =
false)
const;
615 operator std::string()
const {
return toString(); }
GLuint getWrapT() const
Definition: CUTexture.h:473
GLfloat getMaxS() const
Definition: CUTexture.h:570
void setWrapS(GLuint wrap)
Size getSize()
Definition: CUTexture.h:399
const Texture & operator=(const void *data)
Definition: CUTexture.h:288
const std::shared_ptr< Texture > & getParent() const
Definition: CUTexture.h:501
unsigned int getHeight() const
Definition: CUTexture.h:392
void setWrapT(GLuint wrap)
std::shared_ptr< Texture > getParent()
Definition: CUTexture.h:510
GLuint getMinFilter() const
Definition: CUTexture.h:419
std::shared_ptr< Texture > getSubTexture(GLfloat minS, GLfloat maxS, GLfloat minT, GLfloat maxT)
bool initWithData(const void *data, int width, int height, PixelFormat format=PixelFormat::RGBA)
Definition: CUTexture.h:54
GLfloat getMaxT() const
Definition: CUTexture.h:580
PixelFormat getFormat() const
Definition: CUTexture.h:409
bool initWithFile(const std::string &filename)
void setName(std::string name)
Definition: CUTexture.h:368
bool isSubTexture() const
Definition: CUTexture.h:540
GLuint getMagFilter() const
Definition: CUTexture.h:431
bool isReady() const
Definition: CUTexture.h:313
unsigned int getWidth() const
Definition: CUTexture.h:385
void setMagFilter(GLuint magFilter)
GLfloat getMinS() const
Definition: CUTexture.h:550
void setMinFilter(GLuint minFilter)
bool init(int width, int height, PixelFormat format=PixelFormat::RGBA)
PixelFormat
Definition: CUTexture.h:64
static std::shared_ptr< Texture > allocWithData(const void *data, int width, int height, PixelFormat format=PixelFormat::RGBA)
Definition: CUTexture.h:244
~Texture()
Definition: CUTexture.h:141
const std::string & getName() const
Definition: CUTexture.h:378
static std::shared_ptr< Texture > allocWithFile(const std::string &filename)
Definition: CUTexture.h:269
GLuint getBuffer()
Definition: CUTexture.h:358
bool isActive() const
Definition: CUTexture.h:326
std::string toString(bool verbose=false) const
bool hasMipMaps() const
Definition: CUTexture.h:338
const Texture & set(const void *data)
GLuint getWrapS() const
Definition: CUTexture.h:462
GLfloat getMinT() const
Definition: CUTexture.h:560
static std::shared_ptr< Texture > alloc(int width, int height, PixelFormat format=PixelFormat::RGBA)
Definition: CUTexture.h:222