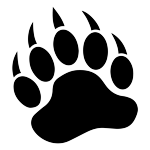 |
CUGL 1.3
Cornell University Game Library
|
49 #ifndef __CU_TEXT_WRITER_H__
50 #define __CU_TEXT_WRITER_H__
51 #include <cugl/base/CUBase.h>
52 #include <cugl/util/CUStrings.h>
53 #include <cugl/io/CUPathname.h>
88 #pragma mark Constructors
121 bool init(
const std::string& file) {
177 bool init(
const std::string& file,
unsigned int capacity) {
195 bool init(
const char* file,
unsigned int capacity) {
218 #pragma mark Static Constructors
235 static std::shared_ptr<TextWriter>
alloc(
const std::string& file) {
236 std::shared_ptr<TextWriter> result = std::make_shared<TextWriter>();
237 return (result->init(file) ? result :
nullptr);
256 static std::shared_ptr<TextWriter>
alloc(
const char* file) {
257 std::shared_ptr<TextWriter> result = std::make_shared<TextWriter>();
258 return (result->init(file) ? result :
nullptr);
278 std::shared_ptr<TextWriter> result = std::make_shared<TextWriter>();
279 return (result->init(file) ? result :
nullptr);
296 static std::shared_ptr<TextWriter>
alloc(
const std::string& file,
unsigned int capacity) {
297 std::shared_ptr<TextWriter> result = std::make_shared<TextWriter>();
298 return (result->init(file,capacity) ? result :
nullptr);
315 static std::shared_ptr<TextWriter>
alloc(
const char* file,
unsigned int capacity) {
316 std::shared_ptr<TextWriter> result = std::make_shared<TextWriter>();
317 return (result->init(file,capacity) ? result :
nullptr);
334 static std::shared_ptr<TextWriter>
alloc(
const Pathname& file,
unsigned int capacity) {
335 std::shared_ptr<TextWriter> result = std::make_shared<TextWriter>();
336 return (result->init(file,capacity) ? result :
nullptr);
341 #pragma mark Stream Management
361 #pragma mark Primitive Methods
462 if (b) {
write(
"true"); }
else {
write(
"false"); }
493 #pragma mark String Methods
503 void write(
const char* s);
514 void write(
const std::string& s);
static std::shared_ptr< TextWriter > alloc(const Pathname &file, unsigned int capacity)
Definition: CUTextWriter.h:334
Uint32 _capacity
Definition: CUTextWriter.h:83
static std::shared_ptr< TextWriter > alloc(const char *file)
Definition: CUTextWriter.h:256
Definition: CUPathname.h:85
bool init(const std::string &file, unsigned int capacity)
Definition: CUTextWriter.h:177
void write(double n)
Definition: CUTextWriter.h:489
bool init(const std::string &file)
Definition: CUTextWriter.h:121
static std::shared_ptr< TextWriter > alloc(const std::string &file, unsigned int capacity)
Definition: CUTextWriter.h:296
static std::shared_ptr< TextWriter > alloc(const char *file, unsigned int capacity)
Definition: CUTextWriter.h:315
void write(Sint16 n)
Definition: CUTextWriter.h:393
static std::shared_ptr< TextWriter > alloc(const Pathname &file)
Definition: CUTextWriter.h:277
static std::shared_ptr< TextWriter > alloc(const std::string &file)
Definition: CUTextWriter.h:235
void write(Uint64 n)
Definition: CUTextWriter.h:448
bool init(const char *file)
Definition: CUTextWriter.h:141
void write(Sint64 n)
Definition: CUTextWriter.h:437
SDL_RWops * _stream
Definition: CUTextWriter.h:78
char * _cbuffer
Definition: CUTextWriter.h:81
void writeLine(const char *s)
void write(Uint32 n)
Definition: CUTextWriter.h:426
void write(float n)
Definition: CUTextWriter.h:476
bool init(const char *file, unsigned int capacity)
Definition: CUTextWriter.h:195
void write(Sint32 n)
Definition: CUTextWriter.h:415
void write(Uint16 n)
Definition: CUTextWriter.h:404
std::string _name
Definition: CUTextWriter.h:76
Sint32 _bufoff
Definition: CUTextWriter.h:85
void write(Uint8 b)
Definition: CUTextWriter.h:382
void write(bool b)
Definition: CUTextWriter.h:461
TextWriter()
Definition: CUTextWriter.h:96
Definition: CUTextWriter.h:73
~TextWriter()
Definition: CUTextWriter.h:103