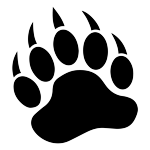 |
CUGL 1.3
Cornell University Game Library
|
42 #ifndef __CU_SPRITE_SHADER_H__
43 #define __CU_SPRITE_SHADER_H__
45 #include <cugl/renderer/CUShader.h>
46 #include <cugl/renderer/CUTexture.h>
47 #include <cugl/math/CUMat4.h>
92 std::shared_ptr<Texture> _mTexture;
95 #pragma mark Constructors
103 _uPerspective(-1), _uTexture(-1) { }
142 bool init(std::string vsource, std::string fsource) {
143 return init(vsource.c_str(),fsource.c_str());
159 bool init(
const char* vsource,
const char* fsource);
162 #pragma mark Static Constructors
173 static std::shared_ptr<SpriteShader>
alloc() {
174 std::shared_ptr<SpriteShader> result = std::make_shared<SpriteShader>();
175 return (result->init() ? result :
nullptr);
191 static std::shared_ptr<SpriteShader>
alloc(std::string vsource, std::string fsource) {
192 std::shared_ptr<SpriteShader> result = std::make_shared<SpriteShader>();
193 return (result->init(vsource, fsource) ? result :
nullptr);
209 static std::shared_ptr<SpriteShader>
alloc(
const char* vsource,
const char* fsource) {
210 std::shared_ptr<SpriteShader> result = std::make_shared<SpriteShader>();
211 return (result->init(vsource, fsource) ? result :
nullptr);
215 #pragma mark Attributes
280 void setTexture(
const std::shared_ptr<Texture>& texture);
294 const std::shared_ptr<Texture>&
getTexture()
const {
return _mTexture; }
297 #pragma mark Rendering
307 void attach(GLuint vArray, GLuint vBuffer);
314 void bind()
override;
324 #pragma mark Compilation
GLint getColorAttr() const
Definition: CUSpriteShader.h:232
GLint getPositionAttr() const
Definition: CUSpriteShader.h:223
bool validateVariable(GLint variable, const char *name)
static std::shared_ptr< SpriteShader > alloc(std::string vsource, std::string fsource)
Definition: CUSpriteShader.h:191
const Mat4 & getPerspective()
Definition: CUSpriteShader.h:273
static std::shared_ptr< SpriteShader > alloc(const char *vsource, const char *fsource)
Definition: CUSpriteShader.h:209
GLint getPerspectiveUni() const
Definition: CUSpriteShader.h:250
bool init(std::string vsource, std::string fsource)
Definition: CUSpriteShader.h:142
void setTexture(const std::shared_ptr< Texture > &texture)
void attach(GLuint vArray, GLuint vBuffer)
SpriteShader()
Definition: CUSpriteShader.h:102
const std::shared_ptr< Texture > & getTexture() const
Definition: CUSpriteShader.h:294
~SpriteShader()
Definition: CUSpriteShader.h:108
Definition: CUSpriteShader.h:74
Definition: CUShader.h:62
GLint getTexCoodAttr() const
Definition: CUSpriteShader.h:241
static std::shared_ptr< SpriteShader > alloc()
Definition: CUSpriteShader.h:173
GLint getTextureUni() const
Definition: CUSpriteShader.h:259
void setPerspective(const Mat4 &matrix)
std::shared_ptr< Texture > getTexture()
Definition: CUSpriteShader.h:287