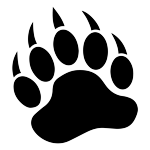 |
CUGL 1.3
Cornell University Game Library
|
47 #ifndef __CU_SLIDER_H__
48 #define __CU_SLIDER_H__
50 #include <cugl/2d/CUNode.h>
51 #include <cugl/2d/CUButton.h>
58 #define DEFAULT_MAX 100
60 #define DEFAULT_RADIUS 20
112 typedef std::function<void(
const std::string& name,
float value)>
Listener;
149 #pragma mark Constructors
175 virtual void dispose()
override;
186 return init(
Vec2(DEFAULT_MIN,DEFAULT_MAX),
Rect(DEFAULT_MIN,DEFAULT_RADIUS,DEFAULT_MAX,0));
230 const std::shared_ptr<Node>& path,
231 const std::shared_ptr<Button>& knob);
260 #pragma mark Static Constructors
270 static std::shared_ptr<Slider>
alloc() {
271 std::shared_ptr<Slider> node = std::make_shared<Slider>();
272 return (node->init() ? node :
nullptr);
293 static std::shared_ptr<Slider>
alloc(
const Vec2& range,
const Rect& bounds) {
294 std::shared_ptr<Slider> node = std::make_shared<Slider>();
295 return (node->init(range,bounds) ? node :
nullptr);
319 const std::shared_ptr<Node>& path,
320 const std::shared_ptr<Button>& knob) {
321 std::shared_ptr<Slider> node = std::make_shared<Slider>();
322 return (node->initWithUI(range,bounds,path,knob) ? node :
nullptr);
349 const std::shared_ptr<JsonValue>& data) {
350 std::shared_ptr<Slider> node = std::make_shared<Slider>();
351 return (node->initWithData(loader,data) ? node :
nullptr);
356 #pragma mark Slider State
449 #pragma mark Appearance
528 #pragma mark Tick Support
588 #pragma mark Listeners
685 #pragma mark Internal Helpers
733 void placeKnob(
const std::shared_ptr<Button>& knob);
745 void placePath(
const std::shared_ptr<Node>& path);
static std::shared_ptr< Slider > alloc()
Definition: CUSlider.h:270
bool _snap
Definition: CUSlider.h:134
Vec2 _range
Definition: CUSlider.h:120
void placePath(const std::shared_ptr< Node > &path)
virtual void dispose() override
void setRange(float min, float max)
Definition: CUSlider.h:427
bool _active
Definition: CUSlider.h:137
std::function< void(const std::string &name, float value)> Listener
Definition: CUSlider.h:112
bool isActive() const
Definition: CUSlider.h:680
float getValue() const
Definition: CUSlider.h:434
bool hasListener() const
Definition: CUSlider.h:599
void placeKnob(const std::shared_ptr< Button > &knob)
bool activate(Uint32 key)
float getMaxValue() const
Definition: CUSlider.h:385
const Vec2 & getRange() const
Definition: CUSlider.h:405
Listener _listener
Definition: CUSlider.h:145
Vec2 _dragpos
Definition: CUSlider.h:141
void setPath(const std::shared_ptr< Node > &path)
Definition: CUSlider.h:495
float _tick
Definition: CUSlider.h:132
void getBounds(const Rect &value)
Definition: CUSlider.h:525
float x
Definition: CUVec2.h:66
std::shared_ptr< Button > _knob
Definition: CUSlider.h:123
bool _mouse
Definition: CUSlider.h:139
void setMinValue(float value)
Definition: CUSlider.h:375
float getTick() const
Definition: CUSlider.h:545
static std::shared_ptr< Slider > alloc(const Vec2 &range, const Rect &bounds)
Definition: CUSlider.h:293
void setListener(Listener listener)
Definition: CUSlider.h:625
virtual bool init() override
Definition: CUSlider.h:185
bool initWithData(const SceneLoader *loader, const std::shared_ptr< JsonValue > &data) override
const std::shared_ptr< Node > & getPath() const
Definition: CUSlider.h:482
Definition: CUSceneLoader.h:77
Definition: CUSlider.h:90
float y
Definition: CUVec2.h:68
bool hasSnap() const
Definition: CUSlider.h:574
void dragKnob(const Vec2 &pos)
Rect _bounds
Definition: CUSlider.h:127
float validate(float value) const
~Slider()
Definition: CUSlider.h:164
static std::shared_ptr< Slider > allocWithUI(const Vec2 &range, const Rect &bounds, const std::shared_ptr< Node > &path, const std::shared_ptr< Button > &knob)
Definition: CUSlider.h:318
void setKnob(const std::shared_ptr< Button > &knob)
Definition: CUSlider.h:472
Rect _adjust
Definition: CUSlider.h:129
float _value
Definition: CUSlider.h:118
const Listener getListener() const
Definition: CUSlider.h:612
const Rect & getBounds() const
Definition: CUSlider.h:510
Uint32 _inputkey
Definition: CUSlider.h:143
void setRange(const Vec2 &range)
Definition: CUSlider.h:415
static std::shared_ptr< Slider > allocWithData(const SceneLoader *loader, const std::shared_ptr< JsonValue > &data)
Definition: CUSlider.h:348
void setTick(float value)
Definition: CUSlider.h:563
void snapTick(bool value)
Definition: CUSlider.h:585
void setMaxValue(float value)
Definition: CUSlider.h:395
void setValue(float value)
Definition: CUSlider.h:445
std::shared_ptr< Node > _path
Definition: CUSlider.h:125
float getMinValue() const
Definition: CUSlider.h:365
const std::shared_ptr< Button > & getKnob() const
Definition: CUSlider.h:458
bool initWithUI(const Vec2 &range, const Rect &bounds, const std::shared_ptr< Node > &path, const std::shared_ptr< Button > &knob)