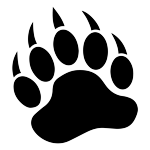 |
CUGL 1.3
Cornell University Game Library
|
41 #ifndef __CU_SCALE_ACTION_H__
42 #define __CU_SCALE_ACTION_H__
71 #pragma mark Constructors
114 return init(factor,0.0f);
129 bool init(
const Vec2& factor,
float time);
131 #pragma mark Static Constructors
139 static std::shared_ptr<ScaleBy>
alloc() {
140 std::shared_ptr<ScaleBy> result = std::make_shared<ScaleBy>();
141 return (result->init() ? result :
nullptr);
154 static std::shared_ptr<ScaleBy>
alloc(
const Vec2& factor) {
155 std::shared_ptr<ScaleBy> result = std::make_shared<ScaleBy>();
156 return (result->init(factor) ? result :
nullptr);
171 static std::shared_ptr<ScaleBy>
alloc(
const Vec2& factor,
float time) {
172 std::shared_ptr<ScaleBy> result = std::make_shared<ScaleBy>();
173 return (result->init(factor,time) ? result :
nullptr);
176 #pragma mark Attributes
198 #pragma mark Animation Methods
204 virtual std::shared_ptr<Action>
clone()
override;
215 virtual void load(
const std::shared_ptr<Node>& target, Uint64* state)
override;
227 virtual void update(
const std::shared_ptr<Node>& target, Uint64* state,
float dt)
override;
229 #pragma mark Debugging Methods
240 virtual std::string
toString(
bool verbose =
false)
const override;
265 #pragma mark Constructors
307 return init(scale,0.0f);
320 bool init(
const Vec2& scale,
float time);
322 #pragma mark Static Constructors
330 static std::shared_ptr<ScaleTo>
alloc() {
331 std::shared_ptr<ScaleTo> result = std::make_shared<ScaleTo>();
332 return (result->init() ? result :
nullptr);
344 static std::shared_ptr<ScaleTo>
alloc(
const Vec2& scale) {
345 std::shared_ptr<ScaleTo> result = std::make_shared<ScaleTo>();
346 return (result->init(scale) ? result :
nullptr);
359 static std::shared_ptr<ScaleTo>
alloc(
const Vec2& scale,
float time) {
360 std::shared_ptr<ScaleTo> result = std::make_shared<ScaleTo>();
361 return (result->init(scale, time) ? result :
nullptr);
364 #pragma mark Attributes
385 #pragma mark Animation Methods
391 virtual std::shared_ptr<Action>
clone()
override;
402 virtual void load(
const std::shared_ptr<Node>& target, Uint64* state)
override;
414 virtual void update(
const std::shared_ptr<Node>& target, Uint64* state,
float dt)
override;
416 #pragma mark Debugging Methods
427 virtual std::string
toString(
bool verbose =
false)
const override;
void dispose()
Definition: CUScaleAction.h:90
static std::shared_ptr< ScaleTo > alloc(const Vec2 &scale, float time)
Definition: CUScaleAction.h:359
Definition: CUAction.h:70
bool init()
Definition: CUScaleAction.h:99
bool init(const Vec2 &factor)
Definition: CUScaleAction.h:113
Vec2 _scale
Definition: CUScaleAction.h:262
~ScaleBy()
Definition: CUScaleAction.h:83
~ScaleTo()
Definition: CUScaleAction.h:277
Vec2 _delta
Definition: CUScaleAction.h:68
static const Vec2 ONE
Definition: CUVec2.h:73
ScaleTo()
Definition: CUScaleAction.h:272
const Vec2 & getFactor() const
Definition: CUScaleAction.h:185
void setScale(const Vec2 &scale)
Definition: CUScaleAction.h:383
bool init()
Definition: CUScaleAction.h:293
virtual void load(const std::shared_ptr< Node > &target, Uint64 *state) override
virtual void update(const std::shared_ptr< Node > &target, Uint64 *state, float dt) override
ScaleBy()
Definition: CUScaleAction.h:78
static std::shared_ptr< ScaleTo > alloc()
Definition: CUScaleAction.h:330
virtual void load(const std::shared_ptr< Node > &target, Uint64 *state) override
static std::shared_ptr< ScaleBy > alloc(const Vec2 &factor)
Definition: CUScaleAction.h:154
static std::shared_ptr< ScaleBy > alloc(const Vec2 &factor, float time)
Definition: CUScaleAction.h:171
virtual std::string toString(bool verbose=false) const override
virtual std::string toString(bool verbose=false) const override
virtual std::shared_ptr< Action > clone() override
static std::shared_ptr< ScaleBy > alloc()
Definition: CUScaleAction.h:139
Definition: CUScaleAction.h:65
virtual std::shared_ptr< Action > clone() override
const Vec2 & getScale() const
Definition: CUScaleAction.h:373
static std::shared_ptr< ScaleTo > alloc(const Vec2 &scale)
Definition: CUScaleAction.h:344
virtual void update(const std::shared_ptr< Node > &target, Uint64 *state, float dt) override
Definition: CUScaleAction.h:259
bool init(const Vec2 &scale)
Definition: CUScaleAction.h:306
void dispose()
Definition: CUScaleAction.h:284
void setFactor(const Vec2 &factor)
Definition: CUScaleAction.h:195