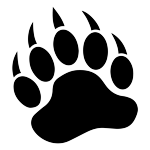 |
CUGL 1.3
Cornell University Game Library
|
41 #ifndef __CU_ROTATE_ACTION_H__
42 #define __CU_ROTATE_ACTION_H__
73 #pragma mark Constructors
102 return init(0.0f, 0.0f);
117 return init(delta, 0.0f);
132 bool init(
float delta,
float time);
134 #pragma mark Static Constructors
142 static std::shared_ptr<RotateBy>
alloc() {
143 std::shared_ptr<RotateBy> result = std::make_shared<RotateBy>();
144 return (result->init() ? result :
nullptr);
158 static std::shared_ptr<RotateBy>
alloc(
float delta) {
159 std::shared_ptr<RotateBy> result = std::make_shared<RotateBy>();
160 return (result->init(delta) ? result :
nullptr);
175 static std::shared_ptr<RotateBy>
alloc(
float delta,
float time) {
176 std::shared_ptr<RotateBy> result = std::make_shared<RotateBy>();
177 return (result->init(delta, time) ? result :
nullptr);
180 #pragma mark Attributes
201 #pragma mark Animation Methods
207 virtual std::shared_ptr<Action>
clone()
override;
219 virtual void update(
const std::shared_ptr<Node>& target, Uint64* state,
float dt)
override;
221 #pragma mark Debugging Methods
232 virtual std::string
toString(
bool verbose =
false)
const override;
237 #pragma mark RotateTo
260 #pragma mark Constructors
291 return init(0.0f, 0.0f);
307 return init(angle, 0.0f);
323 bool init(
float angle,
float time);
325 #pragma mark Static Constructors
335 static std::shared_ptr<RotateTo>
alloc() {
336 std::shared_ptr<RotateTo> result = std::make_shared<RotateTo>();
337 return (result->init() ? result :
nullptr);
352 static std::shared_ptr<RotateTo>
alloc(
float angle) {
353 std::shared_ptr<RotateTo> result = std::make_shared<RotateTo>();
354 return (result->init(angle) ? result :
nullptr);
370 static std::shared_ptr<RotateTo>
alloc(
float angle,
float time) {
371 std::shared_ptr<RotateTo> result = std::make_shared<RotateTo>();
372 return (result->init(angle,time) ? result :
nullptr);
375 #pragma mark Attributes
396 #pragma mark Animation Methods
402 virtual std::shared_ptr<Action>
clone()
override;
413 virtual void load(
const std::shared_ptr<Node>& target, Uint64* state)
override;
425 virtual void update(
const std::shared_ptr<Node>& target, Uint64* state,
float dt)
override;
427 #pragma mark Debugging Methods
438 virtual std::string
toString(
bool verbose =
false)
const override;
Definition: CUAction.h:70
~RotateTo()
Definition: CURotateAction.h:272
float _delta
Definition: CURotateAction.h:70
virtual std::shared_ptr< Action > clone() override
static std::shared_ptr< RotateBy > alloc()
Definition: CURotateAction.h:142
bool init(float delta)
Definition: CURotateAction.h:116
static std::shared_ptr< RotateBy > alloc(float delta, float time)
Definition: CURotateAction.h:175
float _angle
Definition: CURotateAction.h:257
static std::shared_ptr< RotateTo > alloc(float angle, float time)
Definition: CURotateAction.h:370
RotateTo()
Definition: CURotateAction.h:267
void setAngle(float angle)
Definition: CURotateAction.h:394
static std::shared_ptr< RotateTo > alloc(float angle)
Definition: CURotateAction.h:352
bool init()
Definition: CURotateAction.h:290
virtual std::shared_ptr< Action > clone() override
bool init(float angle)
Definition: CURotateAction.h:306
static std::shared_ptr< RotateBy > alloc(float delta)
Definition: CURotateAction.h:158
virtual void update(const std::shared_ptr< Node > &target, Uint64 *state, float dt) override
void dispose()
Definition: CURotateAction.h:279
virtual std::string toString(bool verbose=false) const override
float getDelta() const
Definition: CURotateAction.h:189
bool init()
Definition: CURotateAction.h:101
virtual void load(const std::shared_ptr< Node > &target, Uint64 *state) override
~RotateBy()
Definition: CURotateAction.h:85
RotateBy()
Definition: CURotateAction.h:80
virtual void update(const std::shared_ptr< Node > &target, Uint64 *state, float dt) override
void dispose()
Definition: CURotateAction.h:92
virtual std::string toString(bool verbose=false) const override
float getAngle() const
Definition: CURotateAction.h:384
Definition: CURotateAction.h:67
static std::shared_ptr< RotateTo > alloc()
Definition: CURotateAction.h:335
void setDelta(float delta)
Definition: CURotateAction.h:199
Definition: CURotateAction.h:254