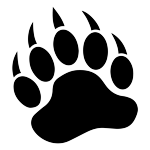 |
CUGL 1.3
Cornell University Game Library
|
60 #pragma mark Constructors
75 Rect(
float x,
float y,
float width,
float height) :
120 Rect&
set(
float x,
float y,
float width,
float height) {
163 #pragma mark Accessors
216 #pragma mark Comparisons
253 bool equals(
const Rect& rect,
float variance=CU_MATH_EPSILON)
const {
402 #pragma mark Rectangle Arithmetic
458 return result.
merge(rect);
492 return result.
expand(factor);
507 return result.
expand(point);
Rect getExpansion(float factor) const
Definition: CURect.h:490
Rect()
Definition: CURect.h:65
bool operator<(const Rect &rect) const
bool equals(const Rect &rect, float variance=CU_MATH_EPSILON) const
Definition: CURect.h:253
Rect(float *array)
Definition: CURect.h:83
Rect & operator=(const float *array)
Definition: CURect.h:106
float getMidX() const
Definition: CURect.h:177
bool operator>=(const Rect &rect) const
Definition: CURect.h:295
Size & set(float width, float height)
Definition: CUSize.h:123
bool touches(const Vec2 &point) const
Rect & intersect(const Rect &rect)
float width
Definition: CUSize.h:61
Rect(const Vec2 &pos, const Size &dimen)
Definition: CURect.h:92
bool operator==(const Rect &rect) const
Definition: CURect.h:226
Rect & expand(float factor)
bool contains(const Rect &rect) const
float x
Definition: CUVec2.h:66
Rect getIntersection(const Rect &rect) const
Definition: CURect.h:472
bool equals(const Vec2 &v, float variance=CU_MATH_EPSILON) const
Definition: CUVec2.h:867
Rect & set(float x, float y, float width, float height)
Definition: CURect.h:120
Vec2 origin
Definition: CURect.h:49
float getMaxY() const
Definition: CURect.h:205
Rect(float x, float y, float width, float height)
Definition: CURect.h:75
Vec2 & set(float x, float y)
Definition: CUVec2.h:153
static const Rect UNIT
Definition: CURect.h:56
float y
Definition: CUVec2.h:68
bool operator>(const Rect &rect) const
Rect & set(const Vec2 &pos, const Size &dimen)
Definition: CURect.h:144
float getMinY() const
Definition: CURect.h:191
bool doesIntersect(const Rect &rect) const
bool inside(const Rect &rect) const
bool operator<=(const Rect &rect) const
Definition: CURect.h:267
Rect & merge(const Rect &rect)
Rect & set(const Rect &other)
Definition: CURect.h:156
Rect & set(const float *array)
Definition: CURect.h:132
Rect getExpansion(const Vec2 &point) const
Definition: CURect.h:505
float getMidY() const
Definition: CURect.h:198
bool equals(const Size &other, float variance=CU_MATH_EPSILON) const
Definition: CUSize.h:301
bool operator!=(const Rect &rect) const
Definition: CURect.h:239
Rect getMerge(const Rect &rect) const
Definition: CURect.h:456
Size size
Definition: CURect.h:51
float isDegenerate() const
Definition: CURect.h:212
float height
Definition: CUSize.h:63
static const Rect ZERO
Definition: CURect.h:54
float getMinX() const
Definition: CURect.h:170
float getMaxX() const
Definition: CURect.h:184