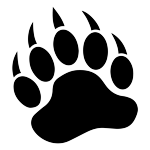 |
CUGL 1.3
Cornell University Game Library
|
46 #ifndef __CU_POLE_ZERO_FIR_H__
47 #define __CU_POLE_ZERO_FIR_H__
49 #include <cugl/math/dsp/CUIIRFilter.h>
50 #include <cugl/math/CUMathBase.h>
51 #include <cugl/util/CUAligned.h>
109 float __attribute__((__aligned__(16))) _c1[4];
110 float __attribute__((__aligned__(16))) _d1[16];
113 float __attribute__((__aligned__(16))) _c2[8];
114 float __attribute__((__aligned__(16))) _d2[16];
123 #pragma mark SPECIALIZED FILTERS
153 void stride(
float gain,
float* input,
float* output,
size_t size,
unsigned channel);
177 void single(
float gain,
float* input,
float* output,
size_t size);
202 void dual(
float gain,
float* input,
float* output,
size_t size);
230 void trio(
float gain,
float* input,
float* output,
size_t size);
255 void quad(
float gain,
float* input,
float* output,
size_t size);
280 void quart(
float gain,
float* input,
float* output,
size_t size);
286 #pragma mark Constructors
313 PoleZeroFIR(
unsigned channels,
float b0,
float b1,
float a1);
334 #pragma mark IIR Signature
372 void setCoeff(
const std::vector<float> &bvals,
const std::vector<float> &avals);
385 const std::vector<float>
getBCoeff()
const;
398 const std::vector<float>
getACoeff()
const;
400 #pragma mark Specialized Attributes
456 #pragma mark Filter Methods
473 void step(
float gain,
float* input,
float* output);
494 void calculate(
float gain,
float* input,
float* output,
size_t size);
509 size_t flush(
float* output);
Definition: CUPoleZeroIIR.h:94
void setAllpass(float coefficient)
const std::vector< float > getACoeff() const
const std::vector< float > getBCoeff() const
void setBlockZero(float pole=0.99f)
unsigned getChannels() const
Definition: CUPoleZeroIIR.h:343
void step(float gain, float *input, float *output)
static bool VECTORIZE
Definition: CUPoleZeroIIR.h:284
void setHighpass(float frequency)
void setChannels(unsigned channels)
void setBCoeff(float b0, float b1)
void setCoeff(const std::vector< float > &bvals, const std::vector< float > &avals)
size_t flush(float *output)
void calculate(float gain, float *input, float *output, size_t size)