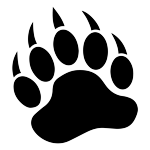 |
CUGL 1.3
Cornell University Game Library
|
45 #ifndef __CU_PHYSICS_WORLD_H__
46 #define __CU_PHYSICS_WORLD_H__
49 #include <Box2D/Dynamics/b2WorldCallbacks.h>
50 #include <cugl/math/cu_math.h>
59 #define DEFAULT_WORLD_STEP 1/60.0f
61 #define DEFAULT_WORLD_VELOC 6
63 #define DEFAULT_WORLD_POSIT 2
67 #pragma mark World Controller
80 class ObstacleWorld :
public b2ContactListener, b2DestructionListener, b2ContactFilter {
96 std::vector<std::shared_ptr<Obstacle>>
_objects;
110 #pragma mark Constructors
167 #pragma mark Static Constructors
181 static std::shared_ptr<ObstacleWorld>
alloc(
const Rect& bounds) {
182 std::shared_ptr<ObstacleWorld> result = std::make_shared<ObstacleWorld>();
183 return (result->init(bounds) ? result :
nullptr);
198 static std::shared_ptr<ObstacleWorld>
alloc(
const Rect& bounds,
const Vec2& gravity) {
199 std::shared_ptr<ObstacleWorld> result = std::make_shared<ObstacleWorld>();
200 return (result->init(bounds,gravity) ? result :
nullptr);
205 #pragma mark Physics Handling
343 #pragma mark Object Management
364 void addObstacle(
const std::shared_ptr<Obstacle>& obj);
407 #pragma mark Collision Callback Functions
469 std::function<void(b2Contact* contact,
const b2Manifold* oldManifold)>
beforeSolve;
488 std::function<void(b2Contact* contact,
const b2ContactImpulse* impulse)>
afterSolve;
539 void PreSolve(b2Contact* contact,
const b2Manifold* oldManifold)
override {
562 void PostSolve(b2Contact* contact,
const b2ContactImpulse* impulse)
override {
570 #pragma mark Filter Callback Functions
603 std::function<bool(b2Fixture* fixtureA, b2Fixture* fixtureB)>
shouldCollide;
624 #pragma mark Destruction Callback Functions
696 #pragma mark Query Functions
705 void queryAABB(std::function<
bool(b2Fixture* fixture)> callback,
const Rect& aabb)
const;
717 void rayCast(std::function<
float(b2Fixture* fixture,
const Vec2& point,
718 const Vec2& normal,
float fraction)> callback,
719 const Vec2& point1,
const Vec2& point2)
const;
bool enabledDestructionCallbacks() const
Definition: CUObstacleWorld.h:644
bool isLockStep() const
Definition: CUObstacleWorld.h:228
Vec2 _gravity
Definition: CUObstacleWorld.h:93
void setVelocityIterations(int velocity)
Definition: CUObstacleWorld.h:273
std::function< void(b2Contact *contact, const b2ContactImpulse *impulse)> afterSolve
Definition: CUObstacleWorld.h:488
bool _lockstep
Definition: CUObstacleWorld.h:85
std::function< void(b2Contact *contact)> onBeginContact
Definition: CUObstacleWorld.h:436
void setGravity(const Vec2 &gravity)
b2World * getWorld()
Definition: CUObstacleWorld.h:219
void setLockStep(bool flag)
Definition: CUObstacleWorld.h:238
void PreSolve(b2Contact *contact, const b2Manifold *oldManifold) override
Definition: CUObstacleWorld.h:539
~ObstacleWorld()
Definition: CUObstacleWorld.h:125
const Vec2 & getGravity() const
Definition: CUObstacleWorld.h:296
static std::shared_ptr< ObstacleWorld > alloc(const Rect &bounds, const Vec2 &gravity)
Definition: CUObstacleWorld.h:198
void addObstacle(const std::shared_ptr< Obstacle > &obj)
void SayGoodbye(b2Fixture *fixture) override
Definition: CUObstacleWorld.h:688
std::function< bool(b2Fixture *fixtureA, b2Fixture *fixtureB)> shouldCollide
Definition: CUObstacleWorld.h:603
bool enabledFilterCallbacks() const
Definition: CUObstacleWorld.h:591
std::vector< std::shared_ptr< Obstacle > > _objects
Definition: CUObstacleWorld.h:96
void activateFilterCallbacks(bool flag)
void setPositionIterations(int position)
Definition: CUObstacleWorld.h:289
static std::shared_ptr< ObstacleWorld > alloc(const Rect &bounds)
Definition: CUObstacleWorld.h:181
void removeObstacle(Obstacle *obj)
void EndContact(b2Contact *contact) override
Definition: CUObstacleWorld.h:512
void SayGoodbye(b2Joint *joint) override
Definition: CUObstacleWorld.h:674
std::function< void(b2Contact *contact)> onEndContact
Definition: CUObstacleWorld.h:446
Definition: CUObstacleWorld.h:80
int _itposition
Definition: CUObstacleWorld.h:91
bool enabledCollisionCallbacks() const
Definition: CUObstacleWorld.h:426
const Rect & getBounds() const
Definition: CUObstacleWorld.h:328
int getPositionIterations() const
Definition: CUObstacleWorld.h:280
void rayCast(std::function< float(b2Fixture *fixture, const Vec2 &point, const Vec2 &normal, float fraction)> callback, const Vec2 &point1, const Vec2 &point2) const
Rect _bounds
Definition: CUObstacleWorld.h:99
int _itvelocity
Definition: CUObstacleWorld.h:89
bool inBounds(Obstacle *obj)
Definition: CUObstacle.h:76
std::function< void(b2Fixture *fixture)> destroyFixture
Definition: CUObstacleWorld.h:654
int getVelocityIterations() const
Definition: CUObstacleWorld.h:264
bool init(const Rect &bounds)
void setStepsize(float step)
Definition: CUObstacleWorld.h:257
b2World * _world
Definition: CUObstacleWorld.h:83
float getStepsize() const
Definition: CUObstacleWorld.h:247
void activateCollisionCallbacks(bool flag)
void queryAABB(std::function< bool(b2Fixture *fixture)> callback, const Rect &aabb) const
void activateDestructionCallbacks(bool flag)
const std::vector< std::shared_ptr< Obstacle > > & getObstacles()
Definition: CUObstacleWorld.h:349
bool ShouldCollide(b2Fixture *fixtureA, b2Fixture *fixtureB) override
Definition: CUObstacleWorld.h:615
std::function< void(b2Contact *contact, const b2Manifold *oldManifold)> beforeSolve
Definition: CUObstacleWorld.h:469
float _stepssize
Definition: CUObstacleWorld.h:87
std::function< void(b2Joint *joint)> destroyJoint
Definition: CUObstacleWorld.h:664
bool _destroy
Definition: CUObstacleWorld.h:106
void PostSolve(b2Contact *contact, const b2ContactImpulse *impulse) override
Definition: CUObstacleWorld.h:562
bool _collide
Definition: CUObstacleWorld.h:102
void BeginContact(b2Contact *contact) override
Definition: CUObstacleWorld.h:498
bool _filters
Definition: CUObstacleWorld.h:104