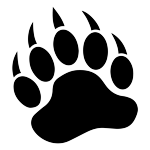 |
CUGL 1.3
Cornell University Game Library
|
36 #ifndef __CU_MP3_DECODER_H__
37 #define __CU_MP3_DECODER_H__
38 #include "CUAudioDecoder.h"
39 #include <codecs/mpg/mpegsound.h>
73 #pragma mark Constructors
96 bool init(
const char* file)
override {
97 return init(std::string(file));
109 bool init(
const std::string& file)
override;
120 #pragma mark Static Constructors
131 static std::shared_ptr<AudioDecoder>
alloc(
const char* file) {
132 return alloc(std::string(file));
145 static std::shared_ptr<AudioDecoder>
alloc(
const std::string& file);
148 #pragma mark Decoding
162 Sint32
pagein(
float* buffer)
override;
173 void setPage(Uint64 page)
override;
void setPage(Uint64 page) override
Mpegtoraw * _decoder
Definition: CUMP3Decoder.h:64
static std::shared_ptr< AudioDecoder > alloc(const char *file)
Definition: CUMP3Decoder.h:131
Definition: CUAudioDecoder.h:55
Soundinputstream _loader
Definition: CUMP3Decoder.h:61
Definition: CUMP3Decoder.h:58
Sint16 * _chunker
Definition: CUMP3Decoder.h:67
Sint32 pagein(float *buffer) override
bool _booted
Definition: CUMP3Decoder.h:70
bool init(const char *file) override
Definition: CUMP3Decoder.h:96