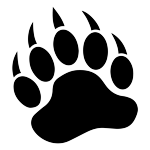 |
CUGL 1.3
Cornell University Game Library
|
48 #ifndef __CU_LOADER_H__
49 #define __CU_LOADER_H__
52 #include <unordered_map>
53 #include <unordered_set>
54 #include <cugl/assets/CUJsonValue.h>
55 #include <cugl/util/CUThreadPool.h>
84 typedef std::function<void(
const std::string& key,
bool success)> LoaderCallback;
87 #pragma mark Polymorphic Base
98 class BaseLoader :
public std::enable_shared_from_this<BaseLoader> {
131 virtual bool read(
const std::string& key,
const std::string& source,
132 LoaderCallback callback,
bool async) {
158 virtual bool read(
const std::shared_ptr<JsonValue>& json,
159 LoaderCallback callback,
bool async) {
176 virtual bool purge(
const std::string& key) {
196 virtual bool purge(
const std::shared_ptr<JsonValue>& json) {
197 return purge(json->key());
211 virtual bool verify(
const std::string& key)
const {
return false; }
215 #pragma mark Constructors
261 return init(
nullptr);
278 virtual bool init(
const std::shared_ptr<ThreadPool>& threads) {
284 #pragma mark AssetManager Support
296 return shared_from_this();
353 #pragma mark Loading/Unloading
370 bool load(
const std::string& key,
const std::string& source) {
371 return read(key,source,
nullptr,
false);
390 bool load(
const char* key,
const std::string& source) {
391 return read(std::string(key),source,
nullptr,
false);
410 bool load(
const std::string& key,
const char* source) {
411 return read(key,std::string(source),
nullptr,
false);
430 bool load(
const char* key,
const char* source) {
431 return read(std::string(key),std::string(source),
nullptr,
false);
455 bool load(
const std::shared_ptr<JsonValue>& json) {
456 return read(json,
nullptr,
false);
478 void loadAsync(
const std::string& key,
const std::string& source, LoaderCallback callback) {
479 read(key, source, callback,
true);
501 void loadAsync(
const char* key,
const std::string& source, LoaderCallback callback) {
502 read(std::string(key), source, callback,
true);
524 void loadAsync(
const std::string& key,
const char* source, LoaderCallback callback) {
525 read(key, std::string(source), callback,
true);
547 void loadAsync(
const char* key,
const char* source, LoaderCallback callback) {
548 read(std::string(key), std::string(source), callback,
true);
575 void loadAsync(
const std::shared_ptr<JsonValue>& json, LoaderCallback callback) {
576 read(json, callback,
true);
626 bool unload(
const std::shared_ptr<JsonValue>& json) {
642 #pragma mark Progress Monitoring
666 return verify(std::string(key));
725 return (size == 0 ? 0.0f : ((
float)
loadCount())/size);
732 #pragma mark Templated Middle Layer
752 std::unordered_map<std::string, std::shared_ptr<T>>
_assets;
771 bool purge(
const std::string& key)
override {
791 bool verify(
const std::string& key)
const override {
796 #pragma mark Constructors
806 #pragma mark Asset Access
817 std::shared_ptr<T>
get(
const std::string& key)
const {
819 return (it ==
_assets.end() ? nullptr : it->second);
832 std::shared_ptr<T>
get(
const char* key)
const {
833 auto it =
_assets.find(std::string(key));
834 return (it ==
_assets.end() ? nullptr : it->second);
847 std::shared_ptr<T>
operator[](
const std::string& key)
const {
return get(key); }
862 #pragma mark Asset Loading
bool load(const char *key, const std::string &source)
Definition: CULoader.h:390
size_t loadCount() const override
Definition: CULoader.h:872
std::shared_ptr< BaseLoader > getHook()
Definition: CULoader.h:295
virtual bool purge(const std::shared_ptr< JsonValue > &json)
Definition: CULoader.h:196
bool complete() const
Definition: CULoader.h:708
void loadAsync(const std::shared_ptr< JsonValue > &json, LoaderCallback callback)
Definition: CULoader.h:575
Definition: CULoader.h:749
AssetManager * _manager
Definition: CULoader.h:112
BaseLoader()
Definition: CULoader.h:222
void setThreadPool(const std::shared_ptr< ThreadPool > &threads)
Definition: CULoader.h:324
virtual bool init(const std::shared_ptr< ThreadPool > &threads)
Definition: CULoader.h:278
std::shared_ptr< ThreadPool > _loader
Definition: CULoader.h:105
bool unload(const std::string &key)
Definition: CULoader.h:592
size_t waitCount() const override
Definition: CULoader.h:884
std::shared_ptr< ThreadPool > getThreadPool() const
Definition: CULoader.h:308
std::shared_ptr< T > get(const std::string &key) const
Definition: CULoader.h:817
bool load(const std::shared_ptr< JsonValue > &json)
Definition: CULoader.h:455
std::shared_ptr< T > operator[](const std::string &key) const
Definition: CULoader.h:847
bool load(const std::string &key, const char *source)
Definition: CULoader.h:410
virtual size_t loadCount() const
Definition: CULoader.h:682
void loadAsync(const char *key, const std::string &source, LoaderCallback callback)
Definition: CULoader.h:501
Loader()
Definition: CULoader.h:803
~BaseLoader()
Definition: CULoader.h:227
void unloadAll() override
Definition: CULoader.h:893
virtual bool read(const std::shared_ptr< JsonValue > &json, LoaderCallback callback, bool async)
Definition: CULoader.h:158
virtual void dispose()
Definition: CULoader.h:243
virtual bool init()
Definition: CULoader.h:260
virtual bool verify(const std::string &key) const
Definition: CULoader.h:211
std::shared_ptr< T > get(const char *key) const
Definition: CULoader.h:832
std::shared_ptr< T > operator[](const char *key) const
Definition: CULoader.h:859
std::unordered_map< std::string, std::shared_ptr< T > > _assets
Definition: CULoader.h:752
virtual void unloadAll()
Definition: CULoader.h:639
bool load(const char *key, const char *source)
Definition: CULoader.h:430
void loadAsync(const std::string &key, const char *source, LoaderCallback callback)
Definition: CULoader.h:524
void loadAsync(const char *key, const char *source, LoaderCallback callback)
Definition: CULoader.h:547
void loadAsync(const std::string &key, const std::string &source, LoaderCallback callback)
Definition: CULoader.h:478
bool load(const std::string &key, const std::string &source)
Definition: CULoader.h:370
const AssetManager * getManager() const
Definition: CULoader.h:348
bool verify(const std::string &key) const override
Definition: CULoader.h:791
std::unordered_set< std::string > _queue
Definition: CULoader.h:755
virtual bool read(const std::string &key, const std::string &source, LoaderCallback callback, bool async)
Definition: CULoader.h:131
bool unload(const std::shared_ptr< JsonValue > &json)
Definition: CULoader.h:626
bool contains(const std::string &key) const
Definition: CULoader.h:652
virtual bool purge(const std::string &key)
Definition: CULoader.h:176
bool purge(const std::string &key) override
Definition: CULoader.h:771
float progress() const
Definition: CULoader.h:723
Definition: CULoader.h:98
virtual size_t waitCount() const
Definition: CULoader.h:697
void setManager(AssetManager *manager)
Definition: CULoader.h:336
Definition: CUAssetManager.h:83
bool unload(const char *key)
Definition: CULoader.h:609
bool contains(const char *key) const
Definition: CULoader.h:665