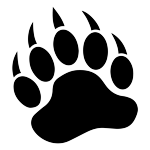 |
CUGL 1.3
Cornell University Game Library
|
32 #ifndef __CU_LAYOUT_H__
33 #define __CU_LAYOUT_H__
34 #include <cugl/2d/CUNode.h>
35 #include <cugl/assets/CUJsonValue.h>
63 #pragma mark Constructors
139 virtual bool init() {
return true; }
152 virtual bool initWithData(
const std::shared_ptr<JsonValue>& data) {
return false; }
172 virtual bool add(
const std::string key,
const std::shared_ptr<JsonValue>& data) {
return false; }
188 virtual bool remove(
const std::string key) {
return false; }
208 #pragma mark Layout Helpers
221 static Anchor getAnchor(
const std::string& x_anchor,
const std::string& y_anchor);
Definition: CULayout.h:61
~Layout()
Definition: CULayout.h:121
static void placeNode(Node *node, Anchor anchor, const Rect &bounds, const Vec2 &offset)
virtual bool init()
Definition: CULayout.h:139
virtual bool remove(const std::string key)
Definition: CULayout.h:188
virtual void layout(Node *node)
Definition: CULayout.h:206
virtual bool initWithData(const std::shared_ptr< JsonValue > &data)
Definition: CULayout.h:152
virtual bool add(const std::string key, const std::shared_ptr< JsonValue > &data)
Definition: CULayout.h:172
Layout()
Definition: CULayout.h:116
virtual void dispose()
Definition: CULayout.h:128
Anchor
Definition: CULayout.h:73
static Anchor getAnchor(const std::string &x_anchor, const std::string &y_anchor)
static void reanchor(Node *node, Anchor anchor)