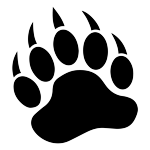 |
CUGL 1.3
Cornell University Game Library
|
39 #ifndef __CU_INPUT_H__
40 #define __CU_INPUT_H__
42 #include <cugl/base/CUBase.h>
43 #include <cugl/util/CUTimestamp.h>
44 #include <unordered_map>
45 #include <unordered_set>
50 #define UINT32_MAX (0xffffffff)
89 std::unordered_map<std::type_index, InputDevice*>
_devices;
92 std::unordered_map<Uint32,std::unordered_set<std::type_index>>
_subscribers;
94 #pragma mark Constructor
105 #pragma mark Application Access
167 bool update(SDL_Event event);
172 #pragma mark Internal Helpers
208 #pragma mark Service Access
219 template <
typename T>
222 std::type_index indx = std::type_index(
typeid(T));
225 return static_cast<T*>(it->second);
240 template <
typename T>
243 std::type_index indx = std::type_index(
typeid(T));
270 template <
typename T>
273 std::type_index indx = std::type_index(
typeid(T));
348 virtual bool init() {
return true; }
388 virtual void queryEvents(std::vector<Uint32>& eventset) = 0;
Definition: CUApplication.h:83
Definition: CUTimestamp.h:61