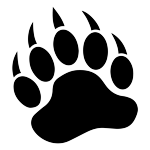 |
CUGL 1.3
Cornell University Game Library
|
46 #ifndef __CU_FONT_LOADER_H__
47 #define __CU_FONT_LOADER_H__
48 #include <cugl/assets/CULoader.h>
49 #include <cugl/2d/CUFont.h>
84 #pragma mark Asset Loading
99 std::shared_ptr<Font>
preload(
const std::string& source,
const std::string& charset,
int size);
118 void materialize(
const std::string& key,
const std::shared_ptr<Font>& font, LoaderCallback callback);
138 bool read(
const std::string& key,
const std::string& source,
139 LoaderCallback callback,
bool async)
override {
162 bool read(
const std::string& key,
const std::string& source,
int size,
163 LoaderCallback callback,
bool async);
189 bool read(
const std::shared_ptr<JsonValue>& json, LoaderCallback callback,
bool async)
override;
194 #pragma mark Constructors
231 static std::shared_ptr<FontLoader>
alloc() {
232 std::shared_ptr<FontLoader> result = std::make_shared<FontLoader>();
233 return (result->init() ? result :
nullptr);
247 static std::shared_ptr<FontLoader>
alloc(
const std::shared_ptr<ThreadPool>& threads) {
248 std::shared_ptr<FontLoader> result = std::make_shared<FontLoader>();
249 return (result->init(threads) ? result :
nullptr);
253 #pragma mark Loading Interface
271 bool load(
const std::string& key,
const std::string& source,
int size) {
272 return read(key, source, size,
nullptr,
false);
292 bool load(
const char* key,
const std::string& source,
int size) {
293 return read(std::string(key), source, size,
nullptr,
false);
313 bool load(
const std::string& key,
const char* source,
int size) {
314 return read(key, std::string(source), size,
nullptr,
false);
334 bool load(
const char* key,
const char* source,
int size) {
335 return read(std::string(key), std::string(source), size,
nullptr,
false);
358 void loadAsync(
const std::string& key,
const std::string& source,
int size,
359 LoaderCallback callback) {
360 read(key, source, size, callback,
true);
383 void loadAsync(
const char* key,
const std::string& source,
int size,
384 LoaderCallback callback) {
385 read(std::string(key), source, size, callback,
true);
408 void loadAsync(
const std::string& key,
const char* source,
int size,
409 LoaderCallback callback) {
410 read(key, std::string(source), size, callback,
true);
433 void loadAsync(
const char* key,
const char* source,
int size,
434 LoaderCallback callback) {
435 read(std::string(key), std::string(source), size, callback,
true);
439 #pragma mark Properties
int getFontSize() const
Definition: CUFontLoader.h:449
Definition: CUFontLoader.h:73
Definition: CULoader.h:749
bool read(const std::string &key, const std::string &source, LoaderCallback callback, bool async) override
Definition: CUFontLoader.h:138
std::shared_ptr< ThreadPool > _loader
Definition: CULoader.h:105
bool load(const char *key, const char *source, int size)
Definition: CUFontLoader.h:334
void loadAsync(const char *key, const char *source, int size, LoaderCallback callback)
Definition: CUFontLoader.h:433
bool load(const char *key, const std::string &source, int size)
Definition: CUFontLoader.h:292
void loadAsync(const std::string &key, const char *source, int size, LoaderCallback callback)
Definition: CUFontLoader.h:408
const std::string & getCharacterSet() const
Definition: CUFontLoader.h:474
static std::shared_ptr< FontLoader > alloc(const std::shared_ptr< ThreadPool > &threads)
Definition: CUFontLoader.h:247
void loadAsync(const char *key, const std::string &source, int size, LoaderCallback callback)
Definition: CUFontLoader.h:383
bool load(const std::string &key, const std::string &source, int size)
Definition: CUFontLoader.h:271
std::unordered_map< std::string, std::shared_ptr< Font > > _assets
Definition: CULoader.h:752
void dispose() override
Definition: CUFontLoader.h:214
void setCharacterSet(const std::string &charset)
Definition: CUFontLoader.h:489
void setDefaultSize(int size)
Definition: CUFontLoader.h:459
int _fontsize
Definition: CUFontLoader.h:80
std::string _charset
Definition: CUFontLoader.h:82
std::shared_ptr< Font > preload(const std::string &source, const std::string &charset, int size)
bool load(const std::string &key, const char *source, int size)
Definition: CUFontLoader.h:313
void loadAsync(const std::string &key, const std::string &source, int size, LoaderCallback callback)
Definition: CUFontLoader.h:358
static std::shared_ptr< FontLoader > alloc()
Definition: CUFontLoader.h:231
void materialize(const std::string &key, const std::shared_ptr< Font > &font, LoaderCallback callback)