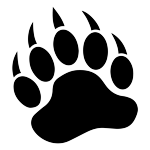 |
CUGL 1.3
Cornell University Game Library
|
46 #ifndef __CU_FLOAT_LAYOUT_H__
47 #define __CU_FLOAT_LAYOUT_H__
48 #include <cugl/2d/layout/CULayout.h>
50 #include <unordered_map>
196 std::unordered_map<std::string,size_t>
_keyset;
203 #pragma mark Internal Helpers
227 #pragma mark Constructors
258 virtual bool initWithData(
const std::shared_ptr<JsonValue>& data)
override;
276 static std::shared_ptr<FloatLayout>
alloc() {
277 std::shared_ptr<FloatLayout> result = std::make_shared<FloatLayout>();
278 return (result->init() ? result :
nullptr);
297 static std::shared_ptr<FloatLayout>
allocWithData(
const std::shared_ptr<JsonValue>& data) {
298 std::shared_ptr<FloatLayout> result = std::make_shared<FloatLayout>();
299 return (result->initWithData(data) ? result :
nullptr);
361 virtual bool add(
const std::string key,
const std::shared_ptr<JsonValue>& data)
override;
380 bool addPriority(
const std::string key,
size_t priority);
396 virtual bool remove(
const std::string key)
override;
void layoutVertical(Node *node)
virtual bool remove(const std::string key) override
Definition: CULayout.h:61
virtual bool initWithData(const std::shared_ptr< JsonValue > &data) override
void setAlignment(Alignment value)
Definition: CUFloatLayout.h:337
void layoutHorizontal(Node *node)
Alignment _alignment
Definition: CUFloatLayout.h:201
Alignment
Definition: CUFloatLayout.h:87
std::unordered_map< std::string, size_t > _keyset
Definition: CUFloatLayout.h:196
static std::shared_ptr< FloatLayout > alloc()
Definition: CUFloatLayout.h:276
bool _horizontal
Definition: CUFloatLayout.h:199
Alignment getAlignment() const
Definition: CUFloatLayout.h:328
virtual void layout(Node *node) override
~FloatLayout()
Definition: CUFloatLayout.h:240
std::vector< std::string > _priority
Definition: CUFloatLayout.h:194
void setHorizontal(bool value)
Definition: CUFloatLayout.h:319
virtual void dispose() override
Definition: CUFloatLayout.h:265
Definition: CUFloatLayout.h:73
virtual bool add(const std::string key, const std::shared_ptr< JsonValue > &data) override
static std::shared_ptr< FloatLayout > allocWithData(const std::shared_ptr< JsonValue > &data)
Definition: CUFloatLayout.h:297
bool isHorizontal() const
Definition: CUFloatLayout.h:310
bool addPriority(const std::string key, size_t priority)