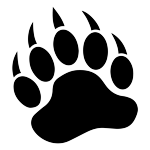 |
CUGL 1.3
Cornell University Game Library
|
42 #ifndef __CU_FADE_ACTION_H__
43 #define __CU_FADE_ACTION_H__
69 #pragma mark Constructors
115 bool init(
float time);
117 #pragma mark Static Constructors
127 static std::shared_ptr<FadeOut>
alloc() {
128 std::shared_ptr<FadeOut> result = std::make_shared<FadeOut>();
129 return (result->init() ? result :
nullptr);
144 static std::shared_ptr<FadeOut>
alloc(
float time) {
145 std::shared_ptr<FadeOut> result = std::make_shared<FadeOut>();
146 return (result->init(time) ? result :
nullptr);
149 #pragma mark Animation Methods
155 virtual std::shared_ptr<Action>
clone()
override;
166 virtual void load(
const std::shared_ptr<Node>& target, Uint64* state)
override;
178 virtual void update(
const std::shared_ptr<Node>& target, Uint64* state,
float dt)
override;
180 #pragma mark Debugging Methods
191 virtual std::string
toString(
bool verbose =
false)
const override;
216 #pragma mark Constructors
262 bool init(
float time);
264 #pragma mark Static Constructors
274 static std::shared_ptr<FadeIn>
alloc() {
275 std::shared_ptr<FadeIn> result = std::make_shared<FadeIn>();
276 return (result->init() ? result :
nullptr);
291 static std::shared_ptr<FadeOut>
alloc(
float time) {
292 std::shared_ptr<FadeOut> result = std::make_shared<FadeOut>();
293 return (result->init(time) ? result :
nullptr);
296 #pragma mark Animation Methods
302 virtual std::shared_ptr<Action>
clone()
override;
313 virtual void load(
const std::shared_ptr<Node>& target, Uint64* state)
override;
325 virtual void update(
const std::shared_ptr<Node>& target, Uint64* state,
float dt)
override;
327 #pragma mark Debugging Methods
338 virtual std::string
toString(
bool verbose =
false)
const override;
~FadeIn()
Definition: CUFadeAction.h:228
Definition: CUAction.h:70
virtual std::shared_ptr< Action > clone() override
virtual void update(const std::shared_ptr< Node > &target, Uint64 *state, float dt) override
virtual std::string toString(bool verbose=false) const override
static std::shared_ptr< FadeIn > alloc()
Definition: CUFadeAction.h:274
virtual void update(const std::shared_ptr< Node > &target, Uint64 *state, float dt) override
void dispose()
Definition: CUFadeAction.h:88
FadeIn()
Definition: CUFadeAction.h:223
virtual void load(const std::shared_ptr< Node > &target, Uint64 *state) override
bool init()
Definition: CUFadeAction.h:246
Definition: CUFadeAction.h:214
virtual std::string toString(bool verbose=false) const override
Definition: CUFadeAction.h:67
FadeOut()
Definition: CUFadeAction.h:76
static std::shared_ptr< FadeOut > alloc(float time)
Definition: CUFadeAction.h:291
~FadeOut()
Definition: CUFadeAction.h:81
virtual std::shared_ptr< Action > clone() override
virtual void load(const std::shared_ptr< Node > &target, Uint64 *state) override
static std::shared_ptr< FadeOut > alloc(float time)
Definition: CUFadeAction.h:144
void dispose()
Definition: CUFadeAction.h:235
static std::shared_ptr< FadeOut > alloc()
Definition: CUFadeAction.h:127
bool init()
Definition: CUFadeAction.h:99