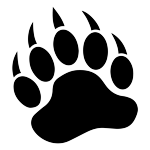 |
CUGL 1.3
Cornell University Game Library
|
49 #ifndef __CU_FIR_FILTER_H__
50 #define __CU_FIR_FILTER_H__
52 #include <cugl/math/dsp/CUIIRFilter.h>
53 #include <cugl/math/CUMathBase.h>
54 #include <cugl/util/CUAligned.h>
111 #pragma mark SPECIALIZED FILTERS
141 void stride(
float gain,
float* input,
float* output,
size_t size,
unsigned channel);
165 void single(
float gain,
float* input,
float* output,
size_t size);
190 void dual(
float gain,
float* input,
float* output,
size_t size);
218 void trio(
float gain,
float* input,
float* output,
size_t size);
243 void quad(
float gain,
float* input,
float* output,
size_t size);
268 void quart(
float gain,
float* input,
float* output,
size_t size);
274 #pragma mark Constructors
299 FIRFilter(
unsigned channels,
const std::vector<float> &bvals);
320 #pragma mark IIR Signature
355 void setCoeff(
const std::vector<float> &bvals,
const std::vector<float> &avals);
368 const std::vector<float>
getBCoeff()
const;
381 const std::vector<float>
getACoeff()
const;
383 #pragma mark Specialized Attributes
395 void setBCoeff(
const std::vector<float> &bvals);
397 #pragma mark Filter Methods
414 void step(
float gain,
float* input,
float* output);
435 void calculate(
float gain,
float* input,
float* output,
size_t size);
452 size_t flush(
float* output);
const std::vector< float > getACoeff() const
Definition: CUFIRFilter.h:92
void setChannels(unsigned channels)
void calculate(float gain, float *input, float *output, size_t size)
unsigned getChannels() const
Definition: CUFIRFilter.h:329
void step(float gain, float *input, float *output)
size_t flush(float *output)
const std::vector< float > getBCoeff() const
void setCoeff(const std::vector< float > &bvals, const std::vector< float > &avals)
static bool VECTORIZE
Definition: CUFIRFilter.h:272
void setBCoeff(const std::vector< float > &bvals)