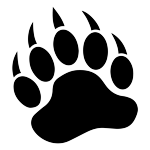 |
CUGL 1.3
Cornell University Game Library
|
43 #ifndef __CU_EASING_BEZIER_H__
44 #define __CU_EASING_BEZIER_H__
46 #include <cugl/math/CUVec2.h>
47 #include "CUEasingFunction.h"
65 class EasingBezier :
public std::enable_shared_from_this<EasingBezier> {
67 #pragma mark Internal Helpers
106 #pragma mark Constructors
163 bool init(
float x1,
float y1,
float x2,
float y2);
182 #pragma mark Static Constructors
188 static std::shared_ptr<EasingBezier>
alloc() {
189 std::shared_ptr<EasingBezier> result = std::make_shared<EasingBezier>();
190 return (result->init() ? result :
nullptr);
204 std::shared_ptr<EasingBezier> result = std::make_shared<EasingBezier>();
205 return (result->init(type) ? result :
nullptr);
222 static std::shared_ptr<EasingBezier>
alloc(
float x1,
float y1,
float x2,
float y2) {
223 std::shared_ptr<EasingBezier> result = std::make_shared<EasingBezier>();
224 return (result->init(x1,y1,x2,y2) ? result :
nullptr);
239 static std::shared_ptr<EasingBezier>
alloc(
const Vec2& p1,
const Vec2& p2) {
240 std::shared_ptr<EasingBezier> result = std::make_shared<EasingBezier>();
241 return (result->init(p1,p2) ? result :
nullptr);
245 #pragma mark Easing Support
void solveCubicEquation(float a, float b, float c, float d)
~EasingBezier()
Definition: CUEasingBezier.h:119
float x
Definition: CUVec2.h:66
Vec2 _c3
Definition: CUEasingBezier.h:74
std::function< float(float)> getEvaluator()
static std::shared_ptr< EasingBezier > alloc(EasingFunction::Type type)
Definition: CUEasingBezier.h:203
std::vector< float > _rootset
Definition: CUEasingBezier.h:77
Definition: CUEasingBezier.h:65
static std::shared_ptr< EasingBezier > alloc(const Vec2 &p1, const Vec2 &p2)
Definition: CUEasingBezier.h:239
Vec2 _c2
Definition: CUEasingBezier.h:72
float y
Definition: CUVec2.h:68
void solveQuadraticEquation(float a, float b, float c)
Vec2 _c1
Definition: CUEasingBezier.h:70
static std::shared_ptr< EasingBezier > alloc()
Definition: CUEasingBezier.h:188
Type
Definition: CUEasingFunction.h:66
static std::shared_ptr< EasingBezier > alloc(float x1, float y1, float x2, float y2)
Definition: CUEasingBezier.h:222
bool init(const Vec2 &p1, const Vec2 &p2)
Definition: CUEasingBezier.h:177
bool init()
Definition: CUEasingBezier.h:133