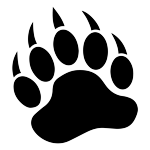 |
CUGL 1.3
Cornell University Game Library
|
40 #ifndef __CU_DELAUNAY_TRIANGULATOR_H__
41 #define __CU_DELAUNAY_TRIANGULATOR_H__
43 #include <cugl/math/CUPoly2.h>
44 #include <cugl/math/CUVec2.h>
45 #include <cugl/math/CUVec3.h>
47 #include <unordered_map>
97 Vertex() : index(-1) {}
105 Vertex(
const Vec2& p, Sint64 i);
116 bool operator==(
const Vertex& v)
const;
127 bool operator!=(
const Vertex& v)
const {
return !(*
this == v); }
139 bool operator<(
const Vertex& v)
const;
151 bool operator> (
const Vertex& v)
const {
return v < *
this; }
163 bool operator<=(
const Vertex& v)
const {
return !(v < *
this); }
175 bool operator>=(
const Vertex& v)
const {
return !(*
this < v); }
188 size_t operator()(
const Vertex& v)
const;
226 Edge(
const Vertex& p1,
const Vertex& p2);
238 bool operator==(
const Edge& t)
const;
250 bool operator!=(
const Edge& t)
const {
return !(*
this == t); }
263 size_t operator()(
const Edge& t)
const;
272 bool hasVertex(
const Vec2& v)
const;
281 bool isDegenerate()
const;
285 #pragma mark Triangle
311 Triangle() : isbad(
true) {}
322 Triangle(
const Vertex& p1,
const Vertex& p2,
const Vertex& p3);
334 bool operator==(
const Triangle& t);
346 bool operator!=(
const Triangle& t) {
return !(*
this == t); }
359 size_t operator()(
const Triangle& t)
const;
368 bool hasVertex(
const Vec2& v);
377 Vec3 getBarycentric(
const Vec2& point)
const;
384 Vec2 getCircleCenter()
const;
391 float getCircleRadius()
const;
400 bool containsInCircle(
const Vec2& point)
const;
407 void setBad(
bool bad);
423 bool isDegenerate()
const;
432 bool isExterior()
const;
436 #pragma mark Delaunay
438 std::vector<Vec2> _input;
440 std::vector<Triangle> _output;
442 std::vector<Vec2> _dual;
444 std::vector<std::vector<Edge>> _voronoi;
484 #pragma mark Initialization
501 _input = poly._vertices;
515 void set(
const std::vector<Vec2>& points) {
524 _calculated =
false; _dualated =
false;
525 _output.clear(); _voronoi.clear();
535 _calculated =
false; _dualated =
false;
536 _input.clear(); _output.clear(); _voronoi.clear();
539 #pragma mark Calculation
556 #pragma mark Materialization
619 #pragma mark Voronoization
705 #pragma mark Internal Data Generation
DelaunayTriangulator(const Poly2 &poly)
Definition: CUDelaunayTriangulator.h:477
Poly2 getVoronoiCell(size_t index) const
std::vector< Poly2 > getVoronoi() const
void sortCell(size_t index, const Rect &rect)
std::vector< unsigned short > getTriangulation() const
Poly2 getVoronoiFrame() const
void clear()
Definition: CUDelaunayTriangulator.h:534
Rect getBoundingBox() const
void computeDelaunay(const Rect &rect)
void reset()
Definition: CUDelaunayTriangulator.h:523
void set(const Poly2 &poly)
Definition: CUDelaunayTriangulator.h:499
Definition: CUPoly2.h:109
void set(const std::vector< Vec2 > &points)
Definition: CUDelaunayTriangulator.h:515
DelaunayTriangulator(const std::vector< Vec2 > &points)
Definition: CUDelaunayTriangulator.h:464
void computeVoronoi(const Rect &rect)
Definition: CUDelaunayTriangulator.h:75
~DelaunayTriangulator()
Definition: CUDelaunayTriangulator.h:482
DelaunayTriangulator()
Definition: CUDelaunayTriangulator.h:454