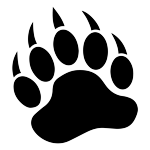 |
CUGL 1.3
Cornell University Game Library
|
38 #ifndef __CU_DECORATOR_NODE_H__
39 #define __CU_DECORATOR_NODE_H__
41 #include <cugl/ai/behavior/CUBehaviorNode.h>
65 #pragma mark Constructors
79 #pragma mark Tree Management
104 template <
typename T>
106 return dynamic_cast<const T*>(
getChild());
123 virtual void query(
float dt)
override;
DecoratorNode()
Definition: CUDecoratorNode.h:72
std::string _classname
Definition: CUBehaviorNode.h:306
virtual BehaviorNode::State update(float dt) override
virtual void query(float dt) override
const BehaviorNode * getChild() const
Definition: CUDecoratorNode.h:89
Definition: CUBehaviorNode.h:280
~DecoratorNode()
Definition: CUDecoratorNode.h:77
State
Definition: CUBehaviorNode.h:290
Definition: CUDecoratorNode.h:63
std::vector< std::shared_ptr< BehaviorNode > > _children
Definition: CUBehaviorNode.h:321