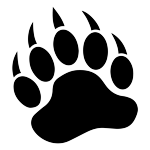 |
CUGL 1.3
Cornell University Game Library
|
37 #ifndef __CU_COMPOSITE_NODE_H__
38 #define __CU_COMPOSITE_NODE_H__
40 #include <cugl/ai/behavior/CUBehaviorNode.h>
70 #pragma mark Internal Helpers
82 #pragma mark Constructors
103 virtual void dispose()
override;
105 #pragma mark Attributes
130 #pragma mark Tree Management
165 template <
typename T>
192 template <
typename T>
197 #pragma mark Behavior Management
212 virtual void query(
float dt)
override;
bool isPreemptive() const
Definition: CUCompositeNode.h:116
BehaviorNode::State update(float dt) override
Definition: CUBehaviorNode.h:280
virtual void query(float dt) override
virtual int selectChild() const =0
const BehaviorNode * getChildByPriorityIndex(Uint32 index) const
~CompositeNode()
Definition: CUCompositeNode.h:94
State
Definition: CUBehaviorNode.h:290
void setPreemptive(bool preemptive)
Definition: CUCompositeNode.h:128
bool _preemptive
Definition: CUCompositeNode.h:68
virtual void dispose() override
const BehaviorNode * getActiveChild() const
Definition: CUCompositeNode.h:64