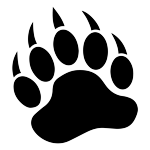 |
CUGL 1.3
Cornell University Game Library
|
45 #ifndef __CU_CAPSULE_OBSTACLE_H__
46 #define __CU_CAPSULE_OBSTACLE_H__
48 #include <Box2D/Collision/Shapes/b2PolygonShape.h>
49 #include <Box2D/Collision/Shapes/b2CircleShape.h>
50 #include <Box2D/Collision/b2Collision.h>
51 #include "CUSimpleObstacle.h"
56 #pragma mark Capsule Obstacle
114 #pragma mark Scene Graph Methods
136 #pragma mark Constructors
157 CUAssertLog(
_core ==
nullptr,
"You must deactive physics before deleting an object");
221 #pragma mark Static Constructors
227 static std::shared_ptr<CapsuleObstacle>
alloc() {
228 std::shared_ptr<CapsuleObstacle> result = std::make_shared<CapsuleObstacle>();
229 return (result->init() ? result :
nullptr);
244 static std::shared_ptr<CapsuleObstacle>
alloc(
const Vec2& pos) {
245 std::shared_ptr<CapsuleObstacle> result = std::make_shared<CapsuleObstacle>();
246 return (result->init(pos) ? result :
nullptr);
266 static std::shared_ptr<CapsuleObstacle>
alloc(
const Vec2& pos,
const Size& size) {
267 std::shared_ptr<CapsuleObstacle> result = std::make_shared<CapsuleObstacle>();
268 return (result->init(pos,size) ? result :
nullptr);
290 std::shared_ptr<CapsuleObstacle> result = std::make_shared<CapsuleObstacle>();
291 return (result->init(pos,size,orient) ? result :
nullptr);
296 #pragma mark Dimensions
378 #pragma mark Physics Methods
412 virtual void setDensity(
float value)
override;
b2PolygonShape _shape
Definition: CUCapsuleObstacle.h:92
float getWidth() const
Definition: CUCapsuleObstacle.h:324
b2CircleShape _ends
Definition: CUCapsuleObstacle.h:94
virtual bool init() override
Definition: CUCapsuleObstacle.h:165
static std::shared_ptr< CapsuleObstacle > alloc(const Vec2 &pos)
Definition: CUCapsuleObstacle.h:244
static std::shared_ptr< CapsuleObstacle > alloc(const Vec2 &pos, const Size &size)
Definition: CUCapsuleObstacle.h:266
void markDirty(bool value)
Definition: CUObstacle.h:811
b2Fixture * _cap1
Definition: CUCapsuleObstacle.h:101
static const Vec2 ZERO
Definition: CUVec2.h:71
const Size & getDimension() const
Definition: CUCapsuleObstacle.h:302
Orientation _orient
Definition: CUCapsuleObstacle.h:107
float width
Definition: CUSize.h:61
float _seamEpsilon
Definition: CUCapsuleObstacle.h:110
const Orientation & getOrientation() const
Definition: CUCapsuleObstacle.h:352
void setDimension(float width, float height)
Definition: CUCapsuleObstacle.h:317
virtual ~CapsuleObstacle()
Definition: CUCapsuleObstacle.h:156
void setWidth(float value)
Definition: CUCapsuleObstacle.h:331
Size _dimension
Definition: CUCapsuleObstacle.h:105
static bool isHorizontal(Orientation value)
Definition: CUCapsuleObstacle.h:373
float getHeight() const
Definition: CUCapsuleObstacle.h:338
virtual void releaseFixtures() override
void setHeight(float value)
Definition: CUCapsuleObstacle.h:345
static std::shared_ptr< CapsuleObstacle > alloc(const Vec2 &pos, const Size &size, Orientation orient)
Definition: CUCapsuleObstacle.h:289
void resize(const Size &size)
b2AABB _center
Definition: CUCapsuleObstacle.h:96
float getSeamOffset() const
Definition: CUCapsuleObstacle.h:401
virtual bool init(const Vec2 &pos) override
Definition: CUCapsuleObstacle.h:179
static std::shared_ptr< CapsuleObstacle > alloc()
Definition: CUCapsuleObstacle.h:227
Definition: CUSimpleObstacle.h:63
void setSeamOffset(float value)
static const Size ZERO
Definition: CUSize.h:66
virtual void setDensity(float value) override
b2Fixture * _core
Definition: CUCapsuleObstacle.h:99
virtual void resetDebug() override
CapsuleObstacle(void)
Definition: CUCapsuleObstacle.h:144
virtual void createFixtures() override
float height
Definition: CUSize.h:63
b2Fixture * _cap2
Definition: CUCapsuleObstacle.h:103
Definition: CUCapsuleObstacle.h:72
Orientation
Definition: CUCapsuleObstacle.h:75
bool setOrientation(Orientation value)
void setDimension(const Size &value)
Definition: CUCapsuleObstacle.h:309