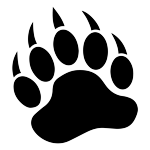 |
CUGL 1.3
Cornell University Game Library
|
38 #ifndef __CU_BEHAVIOR_NODE_H__
39 #define __CU_BEHAVIOR_NODE_H__
40 #include <cugl/ai/behavior/CUBehaviorAction.h>
48 #pragma mark Behavior Node Defintion
186 std::vector<std::shared_ptr<BehaviorNodeDef>>
children;
193 std::shared_ptr<BehaviorActionDef>
action;
213 static std::shared_ptr<BehaviorNodeDef>
alloc() {
214 return std::make_shared<BehaviorNodeDef>();
255 #pragma mark Behavior Node
330 #pragma mark Constructors
354 bool init(
const std::string& name);
365 #pragma mark Attributes
440 virtual std::string
toString(
bool verbose =
false)
const;
445 operator std::string()
const {
return toString(); }
447 #pragma mark Tree Access
500 std::vector<const BehaviorNode*>
getChildren()
const;
528 template <
typename T>
530 return dynamic_cast<const T*>(
getChild(pos));
589 template <
typename T>
612 template <
typename T>
617 #pragma mark Behavior Management
625 virtual void reset();
634 virtual void pause();
657 virtual void start();
673 virtual void query(
float dt) = 0;
706 std::shared_ptr<BehaviorNode>
removeChild(Uint32 pos);
713 void addChild(
const std::shared_ptr<BehaviorNode> child);
727 const std::shared_ptr<BehaviorNode>& b);
bool background
Definition: CUBehaviorNode.h:137
static bool compareSiblings(const std::shared_ptr< BehaviorNode > &a, const std::shared_ptr< BehaviorNode > &b)
std::function< float()> prioritizer
Definition: CUBehaviorNode.h:125
float getPriority() const
Definition: CUBehaviorNode.h:386
virtual std::string toString(bool verbose=false) const
bool uniform
Definition: CUBehaviorNode.h:161
const BehaviorNode * getParent() const
Definition: CUBehaviorNode.h:456
std::string _classname
Definition: CUBehaviorNode.h:306
Type type
Definition: CUBehaviorNode.h:114
std::function< float()> _prioritizer
Definition: CUBehaviorNode.h:318
const BehaviorNode * getNodeByName(const std::string &name) const
std::vector< const BehaviorNode * > getChildren() const
std::shared_ptr< BehaviorNodeDef > getNodeByName(const std::string &name)
Type
Definition: CUBehaviorNode.h:69
std::vector< std::shared_ptr< BehaviorNodeDef > > children
Definition: CUBehaviorNode.h:186
const BehaviorNode * getNodeByName(const char *name) const
Definition: CUBehaviorNode.h:567
void setPrioritizer(const std::function< float()> &func)
Definition: CUBehaviorNode.h:426
float delay
Definition: CUBehaviorNode.h:177
std::string name
Definition: CUBehaviorNode.h:111
int _activeChild
Definition: CUBehaviorNode.h:324
void setPriority(float priority)
void setParent(BehaviorNode *parent)
Definition: CUBehaviorNode.h:466
Definition: CUBehaviorNode.h:280
BehaviorNode::State _state
Definition: CUBehaviorNode.h:312
BehaviorNode * _parent
Definition: CUBehaviorNode.h:309
virtual void query(float dt)=0
std::shared_ptr< BehaviorNode > removeChild(Uint32 pos)
std::shared_ptr< BehaviorNodeDef > getNodeByName(const char *name)
Definition: CUBehaviorNode.h:247
const BehaviorNode * getChild(Uint32 pos) const
std::function< float()> getPrioritizer() const
Definition: CUBehaviorNode.h:415
Definition: CUBehaviorNode.h:57
float _priority
Definition: CUBehaviorNode.h:315
std::string _name
Definition: CUBehaviorNode.h:303
static std::shared_ptr< BehaviorNodeDef > alloc()
Definition: CUBehaviorNode.h:213
int _childOffset
Definition: CUBehaviorNode.h:327
virtual BehaviorNode::State update(float dt)=0
~BehaviorNode()
Definition: CUBehaviorNode.h:342
virtual void setState(BehaviorNode::State state)
bool init(const std::string &name)
size_t getChildCount() const
Definition: CUBehaviorNode.h:489
State
Definition: CUBehaviorNode.h:290
std::shared_ptr< BehaviorActionDef > action
Definition: CUBehaviorNode.h:193
int getParentalOffset() const
Definition: CUBehaviorNode.h:482
BehaviorNode::State getState() const
Definition: CUBehaviorNode.h:395
bool preemptive
Definition: CUBehaviorNode.h:150
void removeFromParent()
Definition: CUBehaviorNode.h:473
void addChild(const std::shared_ptr< BehaviorNode > child)
const std::string & getName() const
Definition: CUBehaviorNode.h:374
std::vector< std::shared_ptr< BehaviorNode > > _children
Definition: CUBehaviorNode.h:321