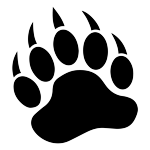 |
CUGL 1.3
Cornell University Game Library
|
39 #ifndef __CU_BEHAVIOR_ACTION_H__
40 #define __CU_BEHAVIOR_ACTION_H__
41 #include <cugl/util/CUDebug.h>
50 #pragma mark Behavior Action Definition
78 std::function<bool(
float dt)>
update;
107 static std::shared_ptr<BehaviorActionDef>
alloc() {
108 return std::make_shared<BehaviorActionDef>();
113 #pragma mark Behavior Action
178 #pragma mark Constructors
199 bool init(
const std::shared_ptr<BehaviorActionDef>& actiondef);
209 #pragma mark Attributes
234 #pragma mark Action Management
std::function< void()> _terminate
Definition: CUBehaviorAction.h:175
BehaviorAction::State _state
Definition: CUBehaviorAction.h:152
std::function< bool(float dt)> update
Definition: CUBehaviorAction.h:78
std::string _name
Definition: CUBehaviorAction.h:149
BehaviorAction::State getState() const
Definition: CUBehaviorAction.h:225
BehaviorAction::State update(float dt)
bool init(const std::shared_ptr< BehaviorActionDef > &actiondef)
std::function< void()> terminate
Definition: CUBehaviorAction.h:87
std::string name
Definition: CUBehaviorAction.h:61
Definition: CUBehaviorAction.h:58
State
Definition: CUBehaviorAction.h:136
std::function< void()> start
Definition: CUBehaviorAction.h:69
BehaviorActionDef()
Definition: CUBehaviorAction.h:98
const std::string & getName() const
Definition: CUBehaviorAction.h:218
void setState(BehaviorAction::State state)
Definition: CUBehaviorAction.h:232
std::function< bool(float dt)> _update
Definition: CUBehaviorAction.h:166
std::function< void()> _start
Definition: CUBehaviorAction.h:159
static std::shared_ptr< BehaviorActionDef > alloc()
Definition: CUBehaviorAction.h:107
~BehaviorAction()
Definition: CUBehaviorAction.h:190
Definition: CUBehaviorAction.h:126