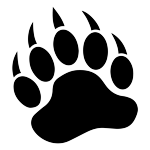 |
CUGL 1.3
Cornell University Game Library
|
38 #ifndef __CU_AUDIO_SAMPLE_H__
39 #define __CU_AUDIO_SAMPLE_H__
41 #include <cugl/assets/CUJsonValue.h>
71 #pragma mark File Types
108 #pragma mark Constructors
134 bool init(
const char* file,
bool stream=
false);
148 bool init(
const std::string& file,
bool stream=
false) {
149 return init(file.c_str(),stream);
165 bool init(Uint8 channels, Uint32 rate, Uint32 frames);
173 virtual void dispose()
override;
201 #pragma mark Static Constructors
214 static std::shared_ptr<AudioSample>
alloc(
const char* file,
bool stream=
false) {
215 std::shared_ptr<AudioSample> result = std::make_shared<AudioSample>();
216 return (result->init(file,stream) ? result :
nullptr);
231 static std::shared_ptr<AudioSample>
alloc(
const std::string& file,
bool stream=
false) {
232 return alloc(file.c_str(), stream);
248 static std::shared_ptr<AudioSample>
alloc(Uint8 channels, Uint32 rate, Uint32 frames) {
249 std::shared_ptr<AudioSample> result = std::make_shared<AudioSample>();
250 return (result->init(channels,rate,frames) ? result :
nullptr);
272 static std::shared_ptr<AudioSample>
allocWithData(
const std::shared_ptr<JsonValue>& data);
275 #pragma mark Attributes
312 #pragma mark Playback Support
333 std::shared_ptr<audio::AudioDecoder>
getDecoder();
344 virtual std::shared_ptr<audio::AudioNode>
createNode()
override;
Definition: CUAudioSample.h:69
Uint32 _rate
Definition: CUSound.h:79
virtual Sint64 getLength() const override
Definition: CUAudioSample.h:301
static Type guessType(const std::string &file)
Definition: CUAudioSample.h:197
static std::shared_ptr< AudioSample > alloc(Uint8 channels, Uint32 rate, Uint32 frames)
Definition: CUAudioSample.h:248
bool _stream
Definition: CUAudioSample.h:102
float * getBuffer()
Definition: CUAudioSample.h:322
float * _buffer
Definition: CUAudioSample.h:105
bool init(const std::string &file, bool stream=false)
Definition: CUAudioSample.h:148
Type
Definition: CUAudioSample.h:79
Uint64 _frames
Definition: CUAudioSample.h:96
static Type guessType(const char *file)
virtual void dispose() override
std::shared_ptr< audio::AudioDecoder > getDecoder()
static std::shared_ptr< AudioSample > alloc(const char *file, bool stream=false)
Definition: CUAudioSample.h:214
Type getType() const
Definition: CUAudioSample.h:292
bool isStreamed() const
Definition: CUAudioSample.h:283
static std::shared_ptr< AudioSample > alloc(const std::string &file, bool stream=false)
Definition: CUAudioSample.h:231
virtual double getDuration() const override
Definition: CUAudioSample.h:310
Type _type
Definition: CUAudioSample.h:99
virtual std::shared_ptr< audio::AudioNode > createNode() override
static std::shared_ptr< AudioSample > allocWithData(const std::shared_ptr< JsonValue > &data)
bool init(const char *file, bool stream=false)
~AudioSample()
Definition: CUAudioSample.h:120