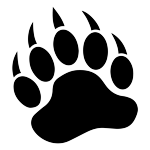 |
CUGL 1.3
Cornell University Game Library
|
44 #ifndef __CU_AUDIO_NODE_H__
45 #define __CU_AUDIO_NODE_H__
93 class AudioNode :
public std::enable_shared_from_this<AudioNode> {
133 typedef std::function<void (
const std::shared_ptr<AudioNode>& node,
Action type)>
Callback;
196 void notify(
const std::shared_ptr<AudioNode>& node,
Action action);
199 #pragma mark Static Attributes
208 #pragma mark Constructors
251 virtual bool init(Uint8 channels, Uint32 rate);
262 #pragma mark Node Attributes
305 virtual void setGain(
float gain);
325 void setName(
const std::string& name);
371 virtual std::string
toString(
bool verbose =
false)
const;
374 operator std::string()
const {
return toString(); }
377 #pragma mark Playback Controls
415 virtual bool pause();
457 virtual Uint32
read(
float* buffer, Uint32 frames);
460 #pragma mark Optional Methods
478 virtual bool mark() {
return false; }
511 virtual bool reset() {
return false; }
528 virtual Sint64
advance(Uint32 frames) {
return -1; }
std::string _localname
Definition: CUAudioNode.h:171
void setName(const char *name)
Definition: CUAudioNode.h:335
std::string _classname
Definition: CUAudioNode.h:136
std::atomic< bool > _calling
Definition: CUAudioNode.h:161
Uint32 _sampling
Definition: CUAudioNode.h:144
Definition: CUAudioNode.h:112
std::atomic< bool > _polling
Definition: CUAudioNode.h:156
void setCallback(Callback callback)
std::function< void(const std::shared_ptr< AudioNode > &node, Action type)> Callback
Definition: CUAudioNode.h:133
virtual void setGain(float gain)
Uint8 getChannels() const
Definition: CUAudioNode.h:272
virtual bool unmark()
Definition: CUAudioNode.h:494
virtual bool reset()
Definition: CUAudioNode.h:511
virtual Sint64 setPosition(Uint32 position)
Definition: CUAudioNode.h:562
size_t _hashOfName
Definition: CUAudioNode.h:181
void setName(const std::string &name)
virtual std::string toString(bool verbose=false) const
Definition: CUAudioNode.h:116
Definition: CUAudioNode.h:108
const static Uint32 DEFAULT_CHANNELS
Definition: CUAudioNode.h:202
virtual Sint64 advance(Uint32 frames)
Definition: CUAudioNode.h:528
bool _booted
Definition: CUAudioNode.h:147
const std::string & getName() const
Definition: CUAudioNode.h:315
virtual Uint32 read(float *buffer, Uint32 frames)
virtual double setRemaining(double time)
Definition: CUAudioNode.h:633
Sint32 getTag() const
Definition: CUAudioNode.h:348
Definition: CUAudioNode.h:118
void notify(const std::shared_ptr< AudioNode > &node, Action action)
virtual Sint64 getPosition() const
Definition: CUAudioNode.h:544
virtual double getRemaining() const
Definition: CUAudioNode.h:614
Uint32 getRate() const
Definition: CUAudioNode.h:283
std::atomic< float > _ndgain
Definition: CUAudioNode.h:150
Uint8 _channels
Definition: CUAudioNode.h:141
virtual double setElapsed(double time)
Definition: CUAudioNode.h:596
Sint32 _tag
Definition: CUAudioNode.h:164
Callback _callback
Definition: CUAudioNode.h:159
std::atomic< bool > _paused
Definition: CUAudioNode.h:153
Action
Definition: CUAudioNode.h:106
const static Uint32 DEFAULT_SAMPLING
Definition: CUAudioNode.h:205
virtual bool completed()
Definition: CUAudioNode.h:437
Definition: CUAudioNode.h:110
void setTag(Sint32 tag)
Definition: CUAudioNode.h:359
Definition: CUAudioNode.h:93
Definition: CUAudioNode.h:114
virtual bool mark()
Definition: CUAudioNode.h:478
virtual double getElapsed() const
Definition: CUAudioNode.h:578