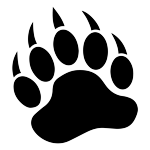 |
CUGL 1.3
Cornell University Game Library
|
46 #ifndef __CU_ANCHORED_LAYOUT_H__
47 #define __CU_ANCHORED_LAYOUT_H__
48 #include <cugl/2d/layout/CULayout.h>
49 #include <unordered_map>
90 std::unordered_map<std::string,Entry>
_entries;
93 #pragma mark Constructors
118 virtual bool initWithData(
const std::shared_ptr<JsonValue>& data)
override {
return true; }
136 static std::shared_ptr<AnchoredLayout>
alloc() {
137 std::shared_ptr<AnchoredLayout> result = std::make_shared<AnchoredLayout>();
138 return (result->init() ? result :
nullptr);
151 static std::shared_ptr<AnchoredLayout>
allocWithData(
const std::shared_ptr<JsonValue>& data) {
152 std::shared_ptr<AnchoredLayout> result = std::make_shared<AnchoredLayout>();
153 return (result->initWithData(data) ? result :
nullptr);
185 virtual bool add(
const std::string key,
const std::shared_ptr<JsonValue>& data)
override;
241 virtual bool remove(
const std::string key)
override;
Definition: CULayout.h:61
virtual void layout(Node *node) override
static std::shared_ptr< AnchoredLayout > alloc()
Definition: CUAnchoredLayout.h:136
bool addAbsolute(const std::string key, Anchor anchor, const Vec2 &offset)
float y_offset
Definition: CUAnchoredLayout.h:82
bool absolute
Definition: CUAnchoredLayout.h:86
~AnchoredLayout()
Definition: CUAnchoredLayout.h:106
Definition: CUAnchoredLayout.h:77
std::unordered_map< std::string, Entry > _entries
Definition: CUAnchoredLayout.h:90
bool addRelative(const std::string key, Anchor anchor, const Vec2 &offset)
float x_offset
Definition: CUAnchoredLayout.h:80
static std::shared_ptr< AnchoredLayout > allocWithData(const std::shared_ptr< JsonValue > &data)
Definition: CUAnchoredLayout.h:151
virtual bool remove(const std::string key) override
virtual void dispose() override
Definition: CUAnchoredLayout.h:125
Anchor anchor
Definition: CUAnchoredLayout.h:84
virtual bool initWithData(const std::shared_ptr< JsonValue > &data) override
Definition: CUAnchoredLayout.h:118
virtual bool add(const std::string key, const std::shared_ptr< JsonValue > &data) override
Definition: CUAnchoredLayout.h:68
Anchor
Definition: CULayout.h:73
AnchoredLayout()
Definition: CUAnchoredLayout.h:101